C#: Determine the type of a particular object
Write a C# Sharp program to determine the type of a particular object.
Sample Solution:-
C# Sharp Code:
using System;
public class Example27
{
public static void Main()
{
// Array containing different data types
object[] values = { (int)24, (long)10653, (byte)24, (sbyte)-5, 26.3, "string" };
// Loop through each value in the 'values' array
foreach (var value in values)
{
// Get the type of the current value
Type t = value.GetType();
// Check the type and print the corresponding message
if (t.Equals(typeof(byte)))
Console.WriteLine("{0} is an unsigned byte.", value);
else if (t.Equals(typeof(sbyte)))
Console.WriteLine("{0} is a signed byte.", value);
else if (t.Equals(typeof(int)))
Console.WriteLine("{0} is a 32-bit integer.", value);
else if (t.Equals(typeof(long)))
Console.WriteLine("{0} is a 64-bit integer.", value); // Fix: Corrected the comment to indicate 64-bit integer
else if (t.Equals(typeof(double)))
Console.WriteLine("{0} is a double-precision floating point.", value);
else
Console.WriteLine("'{0}' is another data type.", value);
}
}
}
Sample Output:
24 is a 32-bit integer. 10653 is a 32-bit integer. 24 is an unsigned byte. -5 is a signed byte. 26.3 is a double-precision floating point. 'string' is another data type.
Flowchart :
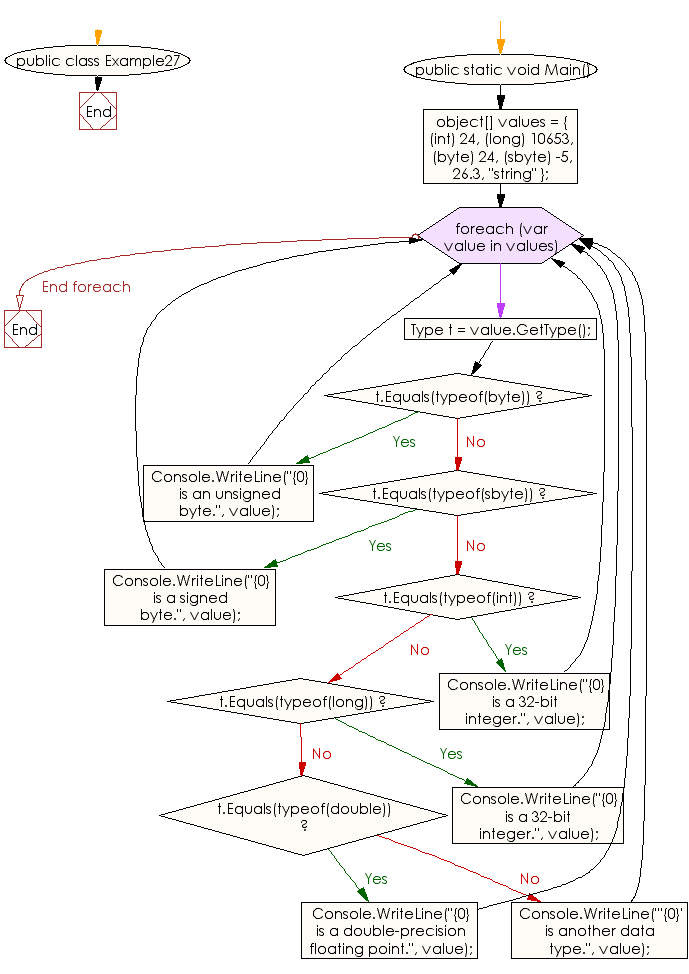
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to display the string representations of a date using the short date format specified for the ja-JP culture.
Next: Write a C# Sharp program to find the leap years between 1994 and 2016.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics