C#: Print all possible standard date and time formats
Write a C# Sharp program to display the string representation of a date using all possible standard date and time formats in the computer's current culture (en-US).
Sample Solution:-
C# Sharp Code:
using System;
public class Example24
{
public static void Main(String[] argv)
{
// Create a DateTime object representing July 28, 2009, at 5:23:15.016 AM
DateTime july28 = new DateTime(2009, 7, 28, 5, 23, 15, 16);
// Get all the possible DateTime formats for the DateTime object 'july28'
string[] july28Formats = july28.GetDateTimeFormats();
// Print out 'july28' in all DateTime formats using the default culture
foreach (string format in july28Formats) {
Console.WriteLine(format);
}
}
}
Sample Output:
7/28/09 07/28/09 07/28/2009 09/07/28 2009-07-28 28-Jul-09 Tuesday, July 28, 2009 July 28, 2009 Tuesday, 28 July, 2009 28 July, 2009 Tuesday, July 28, 2009 5:23 AM Tuesday, July 28, 2009 05:23 AM Tuesday, July 28, 2009 5:23 Tuesday, July 28, 2009 05:23 July 28, 2009 5:23 AM July 28, 2009 05:23 AM July 28, 2009 5:23 July 28, 2009 05:23 Tuesday, 28 July, 2009 5:23 AM Tuesday, 28 July, 2009 05:23 AM -------- Monday, 27 July, 2009 11:53:15 PM Monday, 27 July, 2009 11:53:15 PM Monday, 27 July, 2009 23:53:15 Monday, 27 July, 2009 23:53:15 27 July, 2009 11:53:15 PM 27 July, 2009 11:53:15 PM 27 July, 2009 23:53:15 27 July, 2009 23:53:15 July 2009 July 2009
Flowchart :
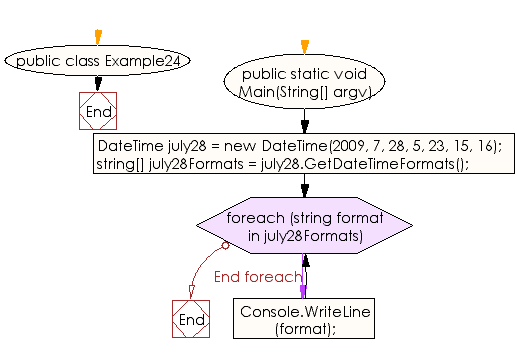
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp Program to convert the specified Windows file time to an equivalent UTC time.
Next: Write a C# Sharp program to display the string representation of a date using the long date format.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.