C#: Long integer to valid time
Write a C# Sharp program which shows that when a time that falls within this range is converted to a long integer value and restored and the original value is adjusted to become a valid time.
Sample Solution:-
C# Sharp Code:
using System;
public class Example22
{
public static void Main()
{
// Create a DateTime object with a potentially invalid time (Daylight Saving Time transition)
DateTime date1 = new DateTime(2016, 3, 14, 2, 30, 00);
// Check if the provided time is an invalid time within the local time zone
Console.WriteLine("Invalid Time: {0}", TimeZoneInfo.Local.IsInvalidTime(date1));
// Convert the DateTime object to a file time format (number of 100-nanosecond intervals since January 1, 1601 UTC)
long ft = date1.ToFileTime();
// Convert the file time back to a DateTime object
DateTime date2 = DateTime.FromFileTime(ft);
// Display the original and reconstructed DateTime objects
Console.WriteLine("{0} -> {1}", date1, date2);
}
}
Sample Output:
Invalid Time: False 3/14/2016 2:30:00 AM -> 3/14/2016 2:30:00 AM
Flowchart :
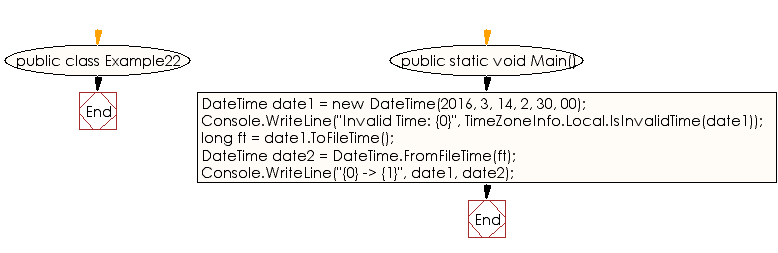
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to convert the specified string representation of a date and time to its DateTime equivalent using the specified array of formats, culture-specific format information, and style.
Next: Write a C# Sharp Program to convert the specified Windows file time to an equivalent UTC time.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.