C#: Demonstrate the Day property
Write a C# Sharp program to display the Day properties (year, month, day, hour, minute, second, millisecond etc.)
Sample Solution:-
C# Sharp Code:
using System;
public class Example2
{
// Main method, entry point of the program
public static void Main()
{
// Creating a DateTime object with specific date and time values
System.DateTime moment = new System.DateTime(2016, 8, 16, 3, 57, 32, 11);
// Displaying the year component of the DateTime object
Console.WriteLine("year = " + moment.Year);
// Displaying the month component of the DateTime object
Console.WriteLine("month = " + moment.Month);
// Displaying the day component of the DateTime object
Console.WriteLine("day = " + moment.Day);
// Displaying the hour component of the DateTime object
Console.WriteLine("hour = " + moment.Hour);
// Displaying the minute component of the DateTime object
Console.WriteLine("minute = " + moment.Minute);
// Displaying the second component of the DateTime object
Console.WriteLine("second = " + moment.Second);
// Displaying the millisecond component of the DateTime object
Console.WriteLine("millisecond = " + moment.Millisecond);
}
}
Sample Output:
year = 2016 month = 8 day = 16 hour = 3 minute = 57 second = 32 millisecond = 11
Flowchart :
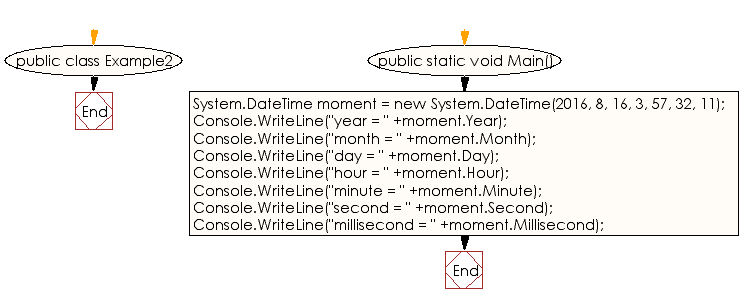
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to extract the Date property and display the DateTime value in the formatted output.
Next: Write a C# Sharp program to get the day of the week for a specified date.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.