C#: Number of days in the specified month and year
Write a C# Sharp program to get the number of days in the specified month and year.
Sample Solution:-
C# Sharp Code:
using System;
public class Example19
{
// Main method, entry point of the program
static void Main()
{
// Constants for months
const int July = 7;
const int Feb = 2;
// Get the number of days in July 2016 (a regular year)
int daysInJuly = System.DateTime.DaysInMonth(2016, July);
Console.WriteLine(daysInJuly);
// Get the number of days in February 2013 (not a leap year)
// The output will be 28 as 2013 was not a leap year
int daysInFeb = System.DateTime.DaysInMonth(2013, Feb);
Console.WriteLine(daysInFeb);
// Get the number of days in February 2004 (a leap year)
// The output will be 29 as 2004 was a leap year
int daysInFebLeap = System.DateTime.DaysInMonth(2004, Feb);
Console.WriteLine(daysInFebLeap);
}
}
Sample Output:
31 28 29
Flowchart:
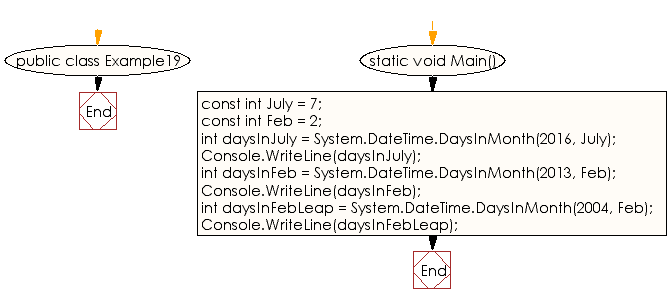
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to compare the current date with a given date.
Next: Write a C# Sharp program to compare DateTime objects.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics