C#: Date of past and future fifteen years of a specified date
Write a C# Sharp program to display the date of the past and future fifteen years from a specified date.
Sample Solution:-
C# Sharp Code:
using System;
public class Example15
{
// Main method, entry point of the program
public static void Main()
{
// Define a base date as February 29, 2016
DateTime baseDate = new DateTime(2016, 2, 29);
// Display the base date
Console.WriteLine(" Base Date: {0:d}\n", baseDate);
// Show dates of previous fifteen years
for (int ctr = -1; ctr >= -15; ctr--)
{
// Calculate and display dates 'ctr' years ago from the base date
Console.WriteLine("{0,2} year(s) ago: {1:d}", Math.Abs(ctr), baseDate.AddYears(ctr));
}
Console.WriteLine(); // Empty line for separation
// Show dates of next fifteen years
for (int ctr = 1; ctr <= 15; ctr++)
{
// Calculate and display dates 'ctr' years from now from the base date
Console.WriteLine("{0,2} year(s) from now: {1:d}", ctr, baseDate.AddYears(ctr));
}
}
}
Sample Output:
Base Date: 2/29/2016 1 year(s) ago: 2/28/2015 2 year(s) ago: 2/28/2014 3 year(s) ago: 2/28/2013 4 year(s) ago: 2/29/2012 5 year(s) ago: 2/28/2011 6 year(s) ago: 2/28/2010 7 year(s) ago: 2/28/2009 8 year(s) ago: 2/29/2008 9 year(s) ago: 2/28/2007 10 year(s) ago: 2/28/2006 11 year(s) ago: 2/28/2005 12 year(s) ago: 2/29/2004 13 year(s) ago: 2/28/2003 14 year(s) ago: 2/28/2002 15 year(s) ago: 2/28/2001 1 year(s) from now: 2/28/2017 2 year(s) from now: 2/28/2018 3 year(s) from now: 2/28/2019 4 year(s) from now: 2/29/2020 5 year(s) from now: 2/28/2021 6 year(s) from now: 2/28/2022 7 year(s) from now: 2/28/2023 8 year(s) from now: 2/29/2024 9 year(s) from now: 2/28/2025 10 year(s) from now: 2/28/2026 11 year(s) from now: 2/28/2027 12 year(s) from now: 2/29/2028 13 year(s) from now: 2/28/2029 14 year(s) from now: 2/28/2030 15 year(s) from now: 2/28/2031
Flowchart:
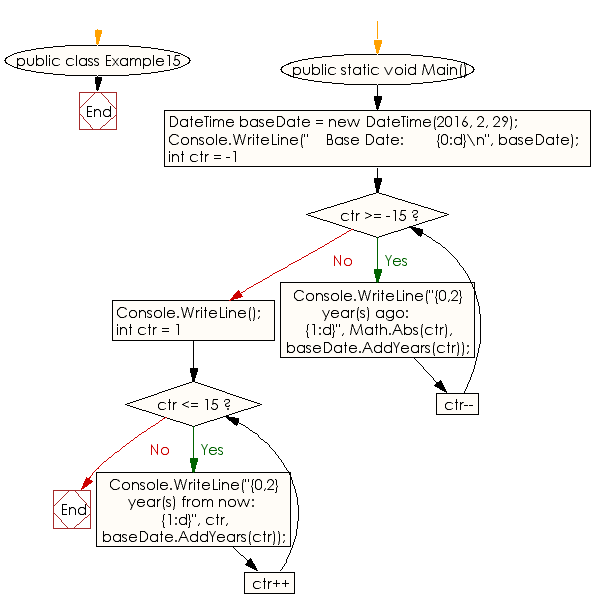
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to add specified number of months between zero and fifteen months to the last day of August, 2016.
Next: Write a C# Sharp program that compares two dates.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.