C#: Add millisecond to a given date
Write a C# Sharp Program to add one millisecond and 2.5 milliseconds to a given date value. Display each value and the difference between it and the original value.
Note: The difference is displayed both as a time span and as a number of ticks and one millisecond equals 10,000 ticks.
Sample Solution:-
C# Sharp Code:
using System;
public class Example12
{
// Main method, entry point of the program
public static void Main()
{
// Date format string for displaying dates with milliseconds
string dateFormat = "MM/dd/yyyy hh:mm:ss.fffffff";
// Create a DateTime object 'date1' set to August 16, 2016, 4:00:00 PM
DateTime date1 = new DateTime(2016, 8, 16, 16, 0, 0);
Console.WriteLine("Original date: {0} ({1:N0} ticks)\n",
date1.ToString(dateFormat), date1.Ticks);
// Create 'date2' by adding 1 millisecond to 'date1'
DateTime date2 = date1.AddMilliseconds(1);
Console.WriteLine("Second date: {0} ({1:N0} ticks)",
date2.ToString(dateFormat), date2.Ticks);
Console.WriteLine("Difference between dates: {0} ({1:N0} ticks)\n",
date2 - date1, date2.Ticks - date1.Ticks);
// Create 'date3' by adding 2.5 milliseconds to 'date1'
DateTime date3 = date1.AddMilliseconds(2.5);
Console.WriteLine("Third date: {0} ({1:N0} ticks)",
date3.ToString(dateFormat), date3.Ticks);
Console.WriteLine("Difference between dates: {0} ({1:N0} ticks)",
date3 - date1, date3.Ticks - date1.Ticks);
}
}
Sample Output:
Original date: 08/16/2016 04:00:00.0000000 (636,069,600,000,000,000 ticks) Second date: 08/16/2016 04:00:00.0010000 (636,069,600,000,010,000 ticks) Difference between dates: 00:00:00.0010000 (10,000 ticks) Third date: 08/16/2016 04:00:00.0030000 (636,069,600,000,030,000 ticks) Difference between dates: 00:00:00.0030000 (30,000 ticks)
Flowchart:
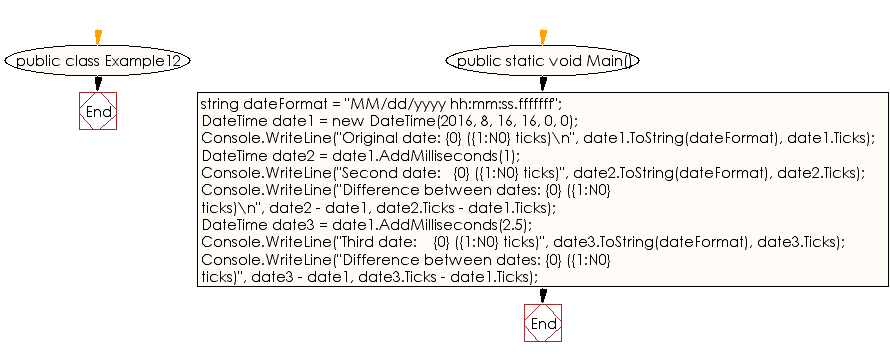
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write C# Sharp program to add a number of whole and fractional values to a date and time.
Next: Write C# Sharp Program to add 30 seconds and the number of seconds in one day to a DateTime value.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.