C#: Calculate and display the surface and volume of a sphere
Write a C# Sharp program that takes the radius of a sphere as input and calculates and displays the surface and volume of the sphere.
C# Sharp: Area and volume of a sphere
A sphere is a perfectly round geometrical object in three-dimensional space that is the surface of a completely round ball.
In three dimensions, the volume inside a sphere is derived to be V = 4/3*π*r3 where r is the radius of the sphere
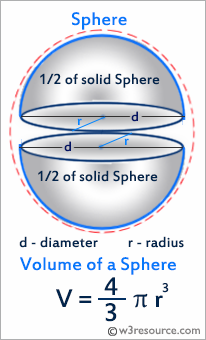
The area of a sphere is A = 4*π*r2
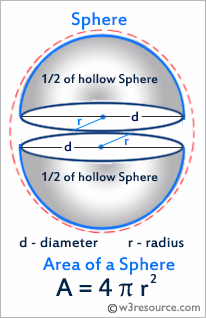
Sample Solution:-
C# Sharp Code:
using System;
public class Exercise8
{
public static void Main()
{
// Declare variable to store the radius of the sphere
float r;
// Define the value of pi
float pi = 3.1415926535f;
// Input the radius of the sphere
Console.Write("Radius: ");
r = Convert.ToSingle(Console.ReadLine());
// Calculate and display the surface area of the sphere
Console.WriteLine("Surface area of the sphere: " + 4 * pi * (r * r));
// Calculate and display the volume of the sphere
Console.WriteLine("Volume of the sphere: " + 4f / 3f * pi * (r * r * r));
}
}
Sample Output:
Radius: 2 50.26548 33.51032
Flowchart:
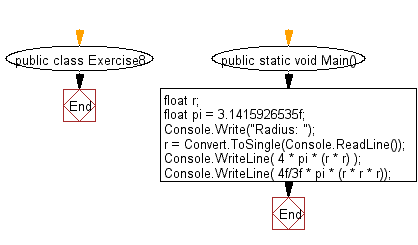
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C# Sharp program that takes distance and time as input and displays the speed in kilometers per hour and miles per hour.
Next: Write a C# Sharp program that takes a character as input and check the input (lowercase) is a vowel, a digit, or any other symbol.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.