C#: Calculate the perimeter and area of a circle
Write a C# Sharp program that takes the radius of a circle as input and calculates the perimeter and area of the circle.
JavaScript: Area and circumference of a circle
In geometry, the area enclosed by a circle of radius r is πr2. Here the Greek letter π represents a constant, approximately equal to 3.14159, which is equal to the ratio of the circumference of any circle to its diameter.
The circumference of a circle is the linear distance around its edge.
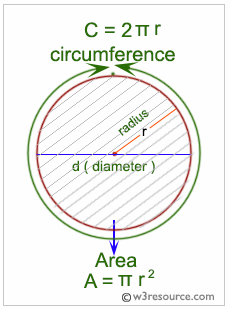
Why is the area of a circle of a circle pi times the square of the radius?
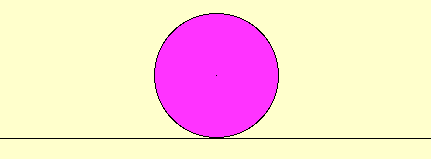
Sample Solution:-
C# Sharp Code:
using System;
public class Exercise5
{
public static void Main()
{
// Declaration of variables
double r, per_cir;
double PI = 3.14; // Constant representing the value of Pi
// Prompting user to input the radius of the circle
Console.WriteLine("Input the radius of the circle : ");
r = Convert.ToDouble(Console.ReadLine()); // Reading user input for radius
// Calculating the perimeter of the circle using the formula: perimeter = 2 * PI * radius
per_cir = 2 * PI * r;
// Displaying the calculated perimeter of the circle
Console.WriteLine("Perimeter of Circle : {0}", per_cir);
Console.Read(); // Keeping console window open until user input to exit
}
}
Sample Output:
Input the radius of the circle : 25 Perimeter of Circle : 157
Flowchart:
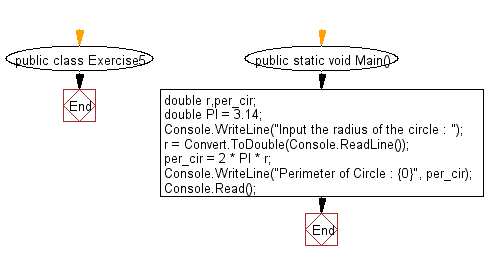
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C# Sharp program that takes two numbers as input and perform an operation (+,-,*,x,/) on them and displays the result of that operation.
Next: Write a C# Sharp program to display certain values of the function x = y2 + 2y + 1 (using integer numbers for y , ranging from -5 to +5).
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics