C#: Decimal to Binary
Write a C# Sharp program that takes a decimal number as input and displays its equivalent in binary form.
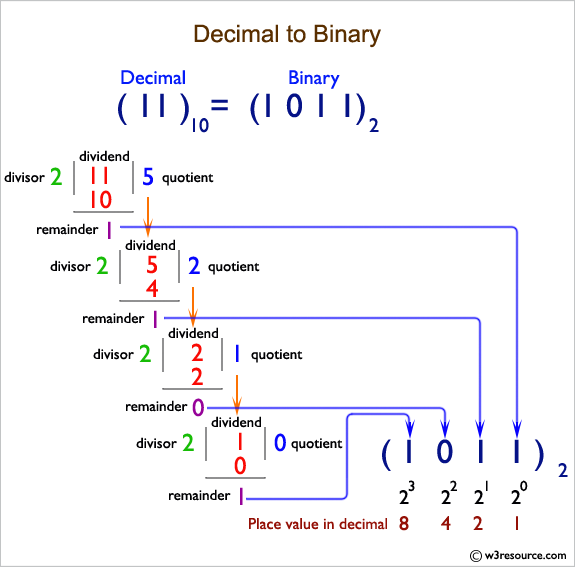
Sample Solution:-
C# Sharp Code:
using System;
public class Exercise10
{
public static void Main()
{
// Declare variables to store two numbers and a boolean to determine if both are even
int n1, n2;
bool bothEven;
// Input the first number
Console.Write("Input First number: ");
n1 = Convert.ToInt32(Console.ReadLine());
// Input the second number
Console.Write("Input Second number: ");
n2 = Convert.ToInt32(Console.ReadLine());
// Check if both numbers are even using logical operators
bothEven = ((n1 % 2 == 0) && (n2 % 2 == 0)) ? true : false;
// Output the result based on whether both numbers are even or not
Console.WriteLine(bothEven ? "There're numbers both even" : "There's a number odd");
}
}
Sample Output:
Input a Number : 65 Binary: 1000001
Flowchart:
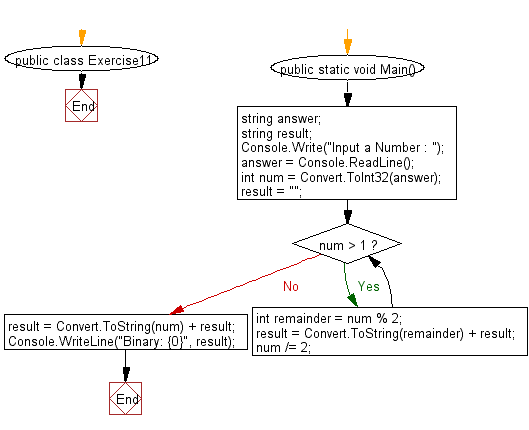
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C# Sharp program that takes two numbers as input and returns true or false when both numbers are even or odd.
Next: C# Sharp Conditional Statement Exercises
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.