C#: Find the quadrant in which the coordinate point lies
Write a C program to accept a coordinate point in an XY coordinate system and determine in which quadrant the coordinate point lies.
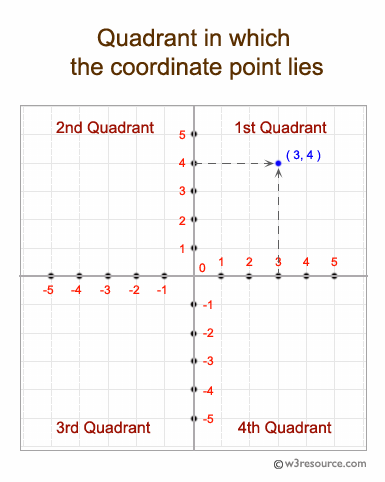
Sample Solution:-
C# Sharp Code:
using System; // Importing the System namespace
public class Exercise9 // Declaration of the Exercise9 class
{
public static void Main() // Entry point of the program
{
int co1, co2; // Declaration of integer variables co1 and co2 for X and Y coordinates
Console.Write("\n\n"); // Printing new lines
Console.Write("Find the quadrant in which the coordinate point lies:\n"); // Displaying the purpose of the program
Console.Write("------------------------------------------------------"); // Displaying a separator
Console.Write("\n\n"); // Printing new lines
Console.Write("Input the value for X coordinate :"); // Prompting user to input the X coordinate
co1 = Convert.ToInt32(Console.ReadLine()); // Reading the input X coordinate from the user
Console.Write("Input the value for Y coordinate :"); // Prompting user to input the Y coordinate
co2 = Convert.ToInt32(Console.ReadLine()); // Reading the input Y coordinate from the user
if (co1 > 0 && co2 > 0) // Checking if X and Y coordinates are both positive
Console.Write("The coordinate point ({0} {1}) lies in the First quadrant.\n\n", co1, co2); // Printing a message for the first quadrant
else if (co1 < 0 && co2 > 0) // Checking if X coordinate is negative and Y coordinate is positive
Console.Write("The coordinate point ({0} {1}) lies in the Second quadrant.\n\n", co1, co2); // Printing a message for the second quadrant
else if (co1 < 0 && co2 < 0) // Checking if both X and Y coordinates are negative
Console.Write("The coordinate point ({0} {1}) lies in the Third quadrant.\n\n", co1, co2); // Printing a message for the third quadrant
else if (co1 > 0 && co2 < 0) // Checking if X coordinate is positive and Y coordinate is negative
Console.Write("The coordinate point ({0} {1}) lies in the Fourth quadrant.\n\n", co1, co2); // Printing a message for the fourth quadrant
else if (co1 == 0 && co2 == 0) // Checking if both X and Y coordinates are zero
Console.Write("The coordinate point ({0} {1}) lies at the origin.\n\n", co1, co2); // Printing a message if the coordinates are at the origin
}
}
Sample Output:
Find the quadrant in which the coordinate point lies: ------------------------------------------------------ Input the value for X coordinate :0 Input the value for Y coordinate :0 The coordinate point (0 0) lies at the origin.
Flowchart:
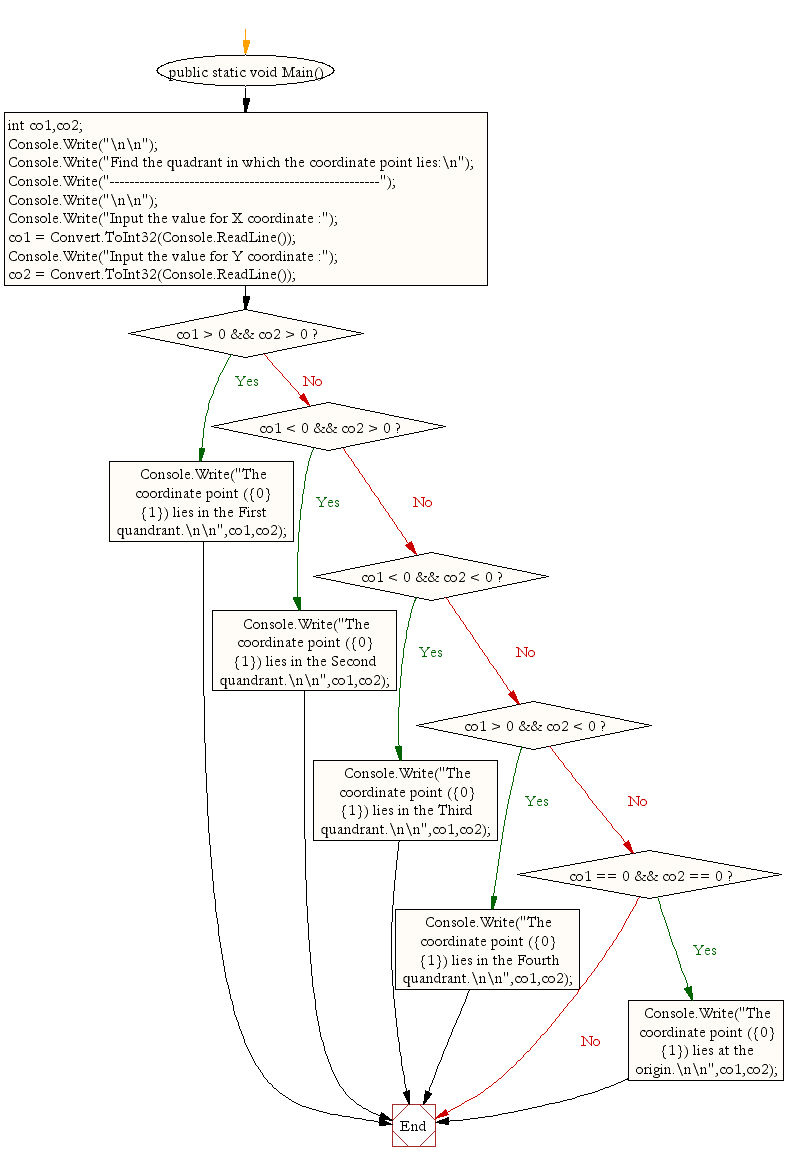
C# Sharp Practice online:
Contribute your code and comments through Disqus.
Previous: Write a C program to find the largest of three numbers.
Next: Write a C program to find the eligibility of admission for a professional course based on the following criteria.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics