C#: Check whether a given year is a leap year or not
Write a C# Sharp program to find out whether a given year is a leap year or not.
Visual Presentation:
Sample Solution:-
C# Sharp Code:
using System; // Importing the System namespace
public class Exercise4 // Declaration of the Exercise4 class
{
public static void Main() // Entry point of the program
{
int chk_year; // Declaration of the integer variable chk_year
Console.Write("\n\n"); // Printing new lines
Console.Write("Check whether a given year is leap year or not:\n"); // Displaying the purpose of the program
Console.Write("----------------------------------------------"); // Displaying a separator
Console.Write("\n\n"); // Printing new lines
Console.Write("Input an year : "); // Prompting user to input a year
chk_year = Convert.ToInt32(Console.ReadLine()); // Reading the input year from the user
if ((chk_year % 400) == 0) // Checking if the year is divisible by 400 without remainder
Console.WriteLine("{0} is a leap year.\n", chk_year); // Printing a message if it's a leap year
else if ((chk_year % 100) == 0) // Checking if the year is divisible by 100 without remainder
Console.WriteLine("{0} is not a leap year.\n", chk_year); // Printing a message if it's not a leap year
else if ((chk_year % 4) == 0) // Checking if the year is divisible by 4 without remainder
Console.WriteLine("{0} is a leap year.\n", chk_year); // Printing a message if it's a leap year
else
Console.WriteLine("{0} is not a leap year.\n", chk_year); // Printing a message if it's not a leap year
}
}
Sample Output:
Check whether a given year is leap year or not: ---------------------------------------------- Input an year : 2017 2017 is not a leap year.
Flowchart :
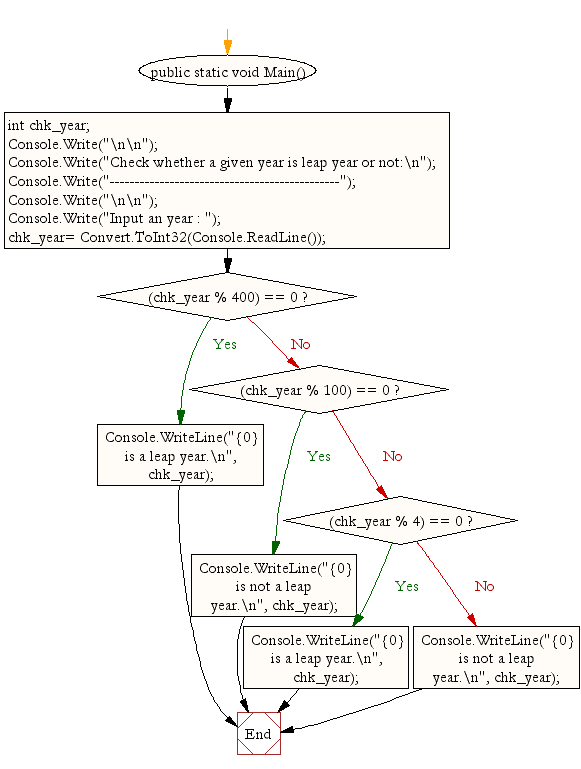
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C# Sharp program to check whether a given number is positive or negative.
Next: Write a C# Sharp program to read age of a candidate and determine whether it is eligible for casting his/her own vote.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.