C#: Check whether a number is positive or negative
Write a C# Sharp program to check whether a given number is positive or negative.
Sample Solution:-
C# Sharp Code:
using System; // Importing the System namespace
public class Exercise3 // Declaration of the Exercise3 class
{
public static void Main() // Entry point of the program
{
int num; // Declaration of the integer variable num
Console.Write("\n\n"); // Printing new lines
Console.Write("Check whether a number is positive or negative:\n"); // Displaying the purpose of the program
Console.Write("----------------------------------------------"); // Displaying a separator
Console.Write("\n\n"); // Printing new lines
Console.Write("Input an integer : "); // Prompting user to input an integer
num = Convert.ToInt32(Console.ReadLine()); // Reading the input integer from the user
if (num >= 0) // Checking if num is greater than or equal to zero
Console.WriteLine("{0} is a positive number.\n", num); // Printing a message if the number is positive
else
Console.WriteLine("{0} is a negative number. \n", num); // Printing a message if the number is negative
}
}
Sample Output:
Check whether a number is positive or negative: ---------------------------------------------- Input an integer : -10 -10 is a negative number.
Pictorial Presentation:
Flowchart:
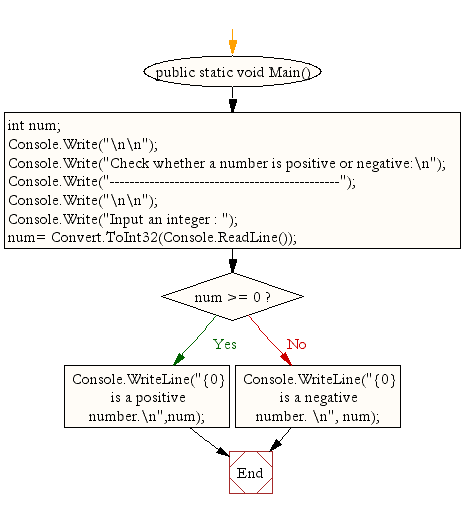
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C program to check whether a given number is even or odd.
Next: Write a C# Sharp program to find whether a given year is a leap year or not.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.