C#: A menu-driven program for a simple calculator
Write a program in C# Sharp which is a menu-driven program to perform simple calculations.
Sample Solution:-
C# Sharp Code:
using System; // Importing necessary namespace
public class Exercise25 // Declaration of the Exercise25 class
{
public static void Main() // Main method, entry point of the program
{
int num1, num2, opt; // Declaration of variables to store integers and user's choice
Console.Write("\n\n"); // Displaying new lines
Console.Write("A menu driven program for a simple calculator:\n"); // Displaying the purpose of the program
Console.Write("------------------------------------------------"); // Displaying a separator
Console.Write("\n\n");
Console.Write("Enter the first Integer :"); // Prompting the user to enter the first integer
num1 = Convert.ToInt32(Console.ReadLine()); // Reading the first integer from user input
Console.Write("Enter the second Integer :"); // Prompting the user to enter the second integer
num2 = Convert.ToInt32(Console.ReadLine()); // Reading the second integer from user input
Console.Write("\nHere are the options :\n"); // Displaying available calculation options
Console.Write("1-Addition.\n2-Subtraction.\n3-Multiplication.\n4-Division.\n5-Exit.\n");
Console.Write("\nInput your choice :"); // Prompting the user to input their choice
opt = Convert.ToInt32(Console.ReadLine()); // Reading the user's choice
switch (opt) // Switch statement based on the user's choice
{
case 1: // Addition
Console.Write("The Addition of {0} and {1} is: {2}\n", num1, num2, num1 + num2);
break;
case 2: // Subtraction
Console.Write("The Subtraction of {0} and {1} is: {2}\n", num1, num2, num1 - num2);
break;
case 3: // Multiplication
Console.Write("The Multiplication of {0} and {1} is: {2}\n", num1, num2, num1 * num2);
break;
case 4: // Division
if (num2 == 0) // Checking if division by zero
{
Console.Write("The second integer is zero. Divide by zero.\n");
}
else
{
Console.Write("The Division of {0} and {1} is : {2}\n", num1, num2, num1 / num2);
}
break;
case 5: // Exit
break;
default: // Handling incorrect option
Console.Write("Input correct option\n");
break;
}
}
}
Sample Output:
A menu driven program for a simple calculator: ------------------------------------------------ Enter the first Integer :20 Enter the second Integer :4 Here are the options : 1-Addition. 2-Substraction. 3-Multiplication. 4-Division. 5-Exit. Input your choice :4 The Division of 20 and 4 is : 5
Flowchart:
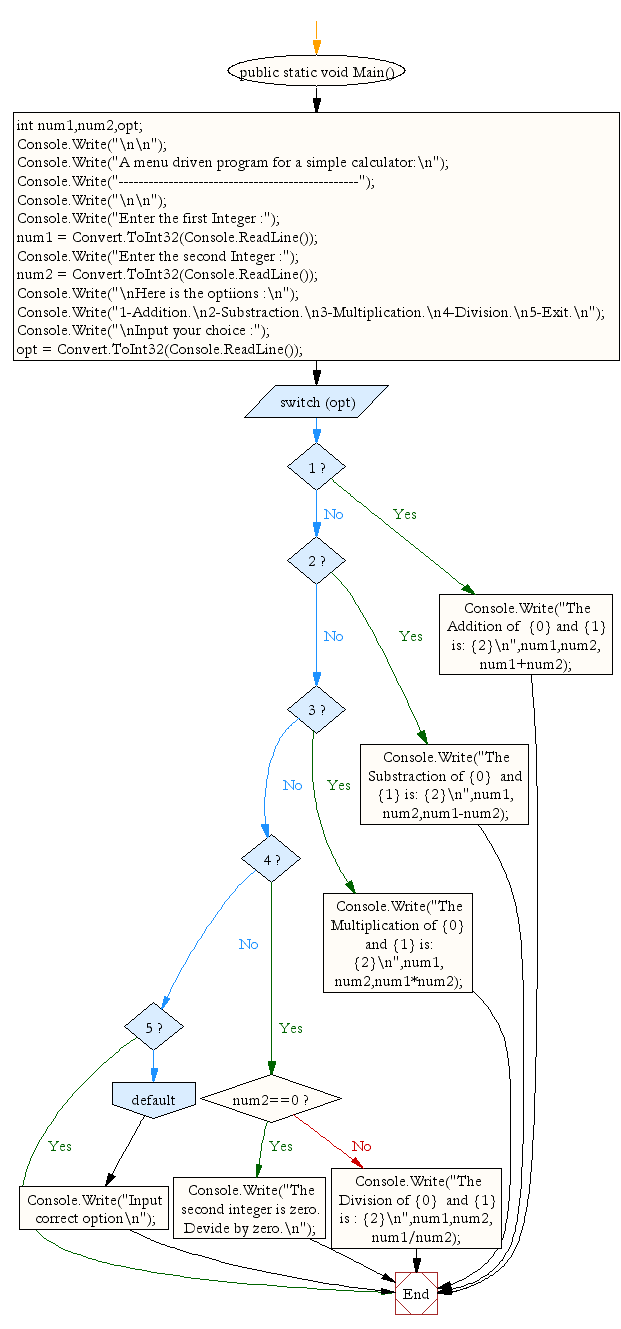
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C# Sharp which is a Menu-Driven Program to compute the area of the various geometrical shape.
Next: C# Sharp programming exercises: For Loop.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.