C#: A menu-driven program to compute the area of the various geometrical shape
Write a C# Sharp program that calculates the area of geometrical shapes using a menu-driven approach.
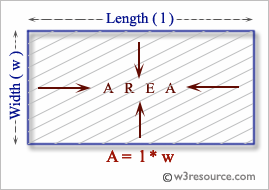
Sample Solution:-
C# Sharp Code:
using System; // Importing necessary namespaces
public class Exercise24 // Declaration of the Exercise24 class
{
public static void Main() // Main method, entry point of the program
{
int choice, r, l, w, b, h; // Declaration of variables for user choices and shape dimensions
double area = 0; // Variable to store the calculated area
Console.Write("\n\n"); // Displaying new lines
Console.Write("A menu driven program to compute the area of various geometrical shapes:\n"); // Displaying the purpose of the program
Console.Write("-------------------------------------------------------------------------"); // Displaying a separator
Console.Write("\n\n");
// Displaying the menu options for calculating the area of different shapes
Console.Write("Input 1 for area of circle\n");
Console.Write("Input 2 for area of rectangle\n");
Console.Write("Input 3 for area of triangle\n");
Console.Write("Input your choice : ");
choice = Convert.ToInt32(Console.ReadLine()); // Reading the user's choice
switch (choice) // Switch statement based on the user's choice
{
case 1: // Calculation for the area of a circle
Console.Write("Input radius of the circle : ");
r = Convert.ToInt32(Console.ReadLine()); // Reading the radius
area = 3.14 * r * r; // Calculating the area of the circle
break;
case 2: // Calculation for the area of a rectangle
Console.Write("Input length of the rectangle : ");
l = Convert.ToInt32(Console.ReadLine()); // Reading the length
Console.Write("Input width of the rectangle : ");
w = Convert.ToInt32(Console.ReadLine()); // Reading the width
area = l * w; // Calculating the area of the rectangle
break;
case 3: // Calculation for the area of a triangle
Console.Write("Input the base of the triangle :");
b = Convert.ToInt32(Console.ReadLine()); // Reading the base
Console.Write("Input the height of the triangle :");
h = Convert.ToInt32(Console.ReadLine()); // Reading the height
area = 0.5 * b * h; // Calculating the area of the triangle
break;
}
Console.Write("The area is : {0}\n", area); // Displaying the calculated area
}
}
Sample Output:
A menu driven program to compute the area of various geometrical shape: ------------------------------------------------------------------------- Input 1 for area of circle Input 2 for area of rectangle Input 3 for area of triangle Input your choice : 2 Input length of the rectangle : 20 Input width of the rectangle : 30 The area is : 600
Flowchart:
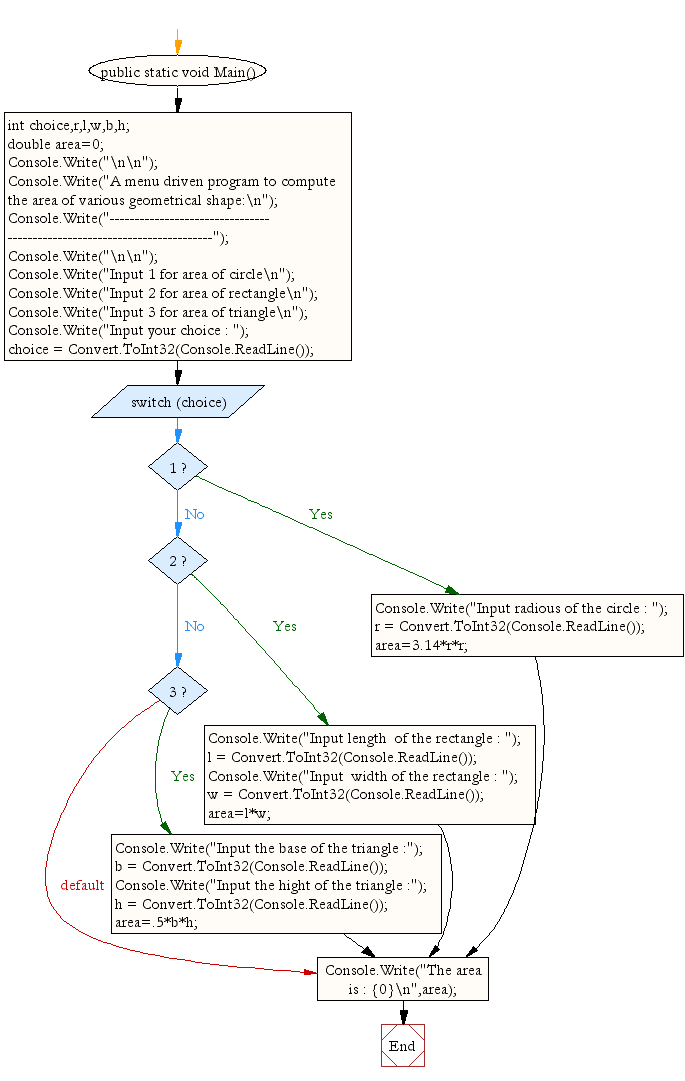
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C# Sharp to read any Month Number in integer and display the number of days for this month.
Next: Write a program in C# Sharp which is a Menu-Driven Program to perform a simple calculation.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.