C#: Read month number and display number of days for that month
Write a program in C# Sharp to read any Month Number in integer and display the number of days for this month.
Sample Solution:-
C# Sharp Code:
using System; // Importing necessary namespaces
public class exercise23 // Declaration of the exercise23 class
{
static void Main(string[] args) // Main method, entry point of the program
{
int monno; // Declaration of a variable to store the month number
Console.Write("\n\n"); // Displaying new lines
Console.Write("Read month number and display number of days for that month:\n"); // Displaying the purpose of the program
Console.Write("--------------------------------------------------------------"); // Displaying a separator
Console.Write("\n\n");
Console.Write("Input Month No : "); // Prompting user to input a month number
monno = Convert.ToInt32(Console.ReadLine()); // Reading the input and converting it to an integer
switch(monno) // Switch statement based on the entered month number
{
case 1:
case 3:
case 5:
case 7:
case 8:
case 10:
case 12:
Console.Write("Month has 31 days. \n"); // Displaying that months 1, 3, 5, 7, 8, 10, and 12 have 31 days
break;
case 2:
Console.Write("The 2nd month is February and has 28 days. \n"); // Displaying that February has 28 days
Console.Write("In leap years, February has 29 days.\n"); // Mentioning that in leap years, February has 29 days
break;
case 4:
case 6:
case 9:
case 11:
Console.Write("Month has 30 days. \n"); // Displaying that months 4, 6, 9, and 11 have 30 days
break;
default:
Console.Write("Invalid Month number.\nPlease try again ....\n"); // Handling invalid month numbers
break;
}
}
}
Sample Output:
Read month number and display number of days for that month: -------------------------------------------------------------- Input Month No : 2 The 2nd month is a February and have 28 days. in leap year The February month Have 29 days.
Visual Presentation:
Flowchart:
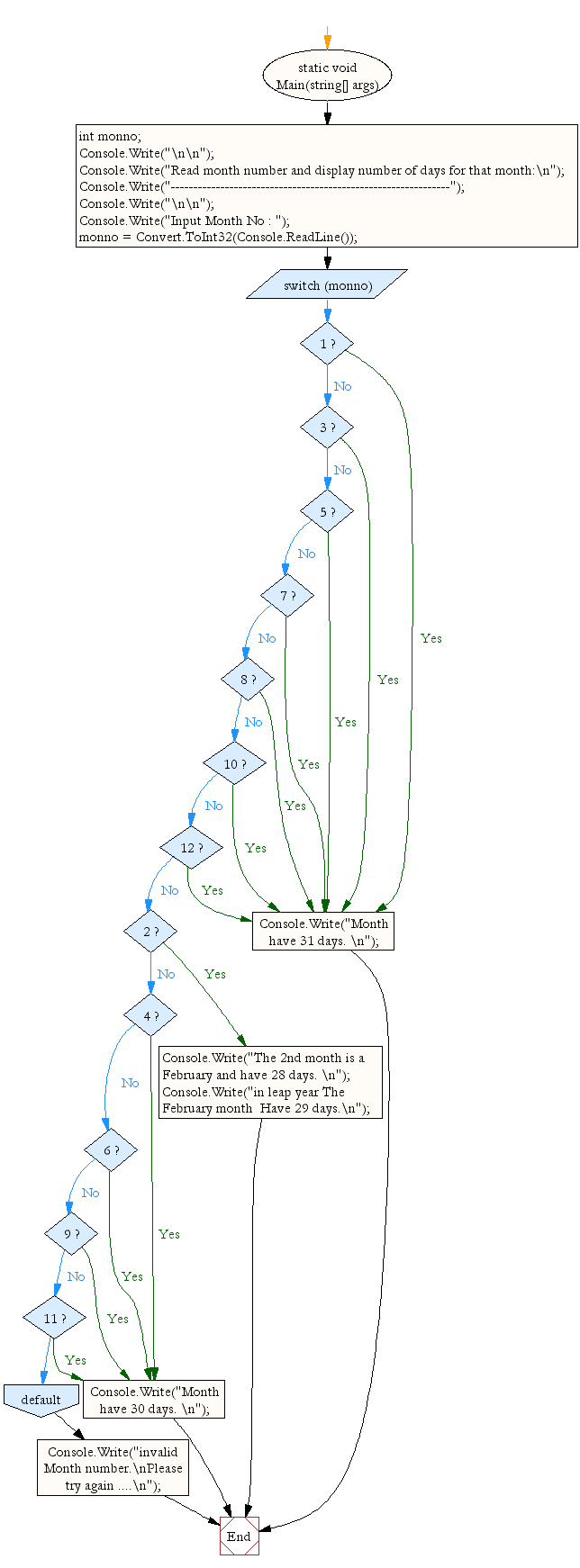
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C# Sharp to read any Month Number in integer and display Month name in the word.
Next: Write a program in C# Sharp which is a Menu-Driven Program to compute the area of the various geometrical shape.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics