C#: Read month number and display month name
Write C# Sharp program to read any Month Number in integer and display Month name.
Sample Solution:-
C# Sharp Code:
using System; // Importing necessary namespaces
public class Exercise22 // Declaration of the Exercise22 class
{
public static void Main() // Main method, entry point of the program
{
int monno; // Declaration of a variable to store the month number
Console.Write("\n\n"); // Displaying new lines
Console.Write("Read month number and display month name:\n"); // Displaying the purpose of the program
Console.Write("-------------------------------------------"); // Displaying a separator
Console.Write("\n\n");
Console.Write("Input Month No : "); // Prompting user to input a month number
monno = Convert.ToInt32(Console.ReadLine()); // Reading the input and converting it to an integer
switch(monno) // Switch statement based on the entered month number
{
case 1:
Console.Write("January\n"); // Displaying "January" for month number 1
break;
case 2:
Console.Write("February\n"); // Displaying "February" for month number 2
break;
case 3:
Console.Write("March\n"); // Displaying "March" for month number 3
break;
case 4:
Console.Write("April\n"); // Displaying "April" for month number 4
break;
case 5:
Console.Write("May\n"); // Displaying "May" for month number 5
break;
case 6:
Console.Write("June\n"); // Displaying "June" for month number 6
break;
case 7:
Console.Write("July\n"); // Displaying "July" for month number 7
break;
case 8:
Console.Write("August\n"); // Displaying "August" for month number 8
break;
case 9:
Console.Write("September\n"); // Displaying "September" for month number 9
break;
case 10:
Console.Write("October\n"); // Displaying "October" for month number 10
break;
case 11:
Console.Write("November\n"); // Displaying "November" for month number 11
break;
case 12:
Console.Write("December\n"); // Displaying "December" for month number 12
break;
default:
Console.Write("Invalid Month number. \nPlease try again ....\n"); // Handling invalid month numbers
break;
}
}
}
Sample Output:
Read month number and display month name: ------------------------------------------- Input Month No : 2 February
Visual Presentation:
Flowchart:
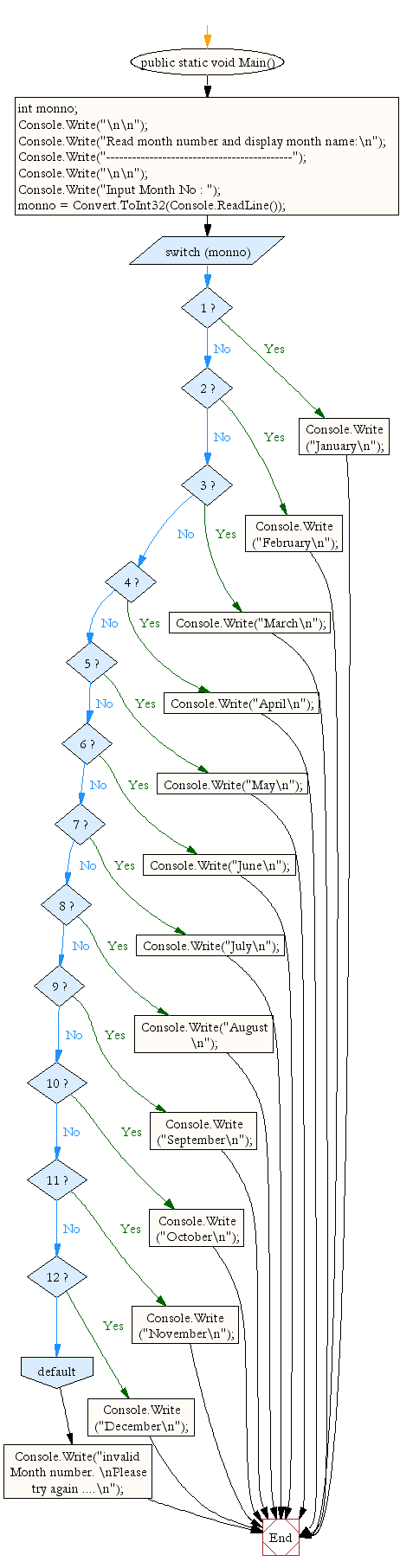
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C# Sharp to read any digit, display in the word.
Next: Write a program in C# Sharp to read any Month Number in integer and display the number of days for this month.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.