C# Sharp Exercises: Accept day number and display its equivalent day name in the word
Write a C# Sharp program to read any day number as an integer and display the name of the day as a word.
Sample Solution:-
C# Sharp Code:
using System; // Importing necessary namespaces
public class Exercise20 // Declaration of the Exercise20 class
{
public static void Main() // Main method, entry point of the program
{
int dayno; // Declaration of a variable to store the day number
Console.Write("\n\n"); // Printing new lines
Console.Write("Accept day number and display its equivalent day name in word:\n"); // Displaying the purpose of the program
Console.Write("----------------------------------------------------------------"); // Displaying a separator
Console.Write("\n\n");
Console.Write("Input Day No : "); // Prompting user to input a day number
dayno = Convert.ToInt32(Console.ReadLine()); // Reading the input and converting it to an integer
switch(dayno) // Switch statement based on the entered day number
{
case 1:
Console.Write("Monday \n"); // Displaying "Monday" for day number 1
break;
case 2:
Console.Write("Tuesday \n"); // Displaying "Tuesday" for day number 2
break;
case 3:
Console.Write("Wednesday \n"); // Displaying "Wednesday" for day number 3
break;
case 4:
Console.Write("Thursday \n"); // Displaying "Thursday" for day number 4
break;
case 5:
Console.Write("Friday \n"); // Displaying "Friday" for day number 5
break;
case 6:
Console.Write("Saturday \n"); // Displaying "Saturday" for day number 6
break;
case 7:
Console.Write("Sunday \n"); // Displaying "Sunday" for day number 7
break;
default:
Console.Write("Invalid day number. \nPlease try again ....\n"); // Handling invalid day numbers
break;
}
}
}
Sample Output:
Accept day number and display its equivalent day name in word: ---------------------------------------------------------------- Input Day No : 5 Friday
Visual Presentation:
Flowchart:
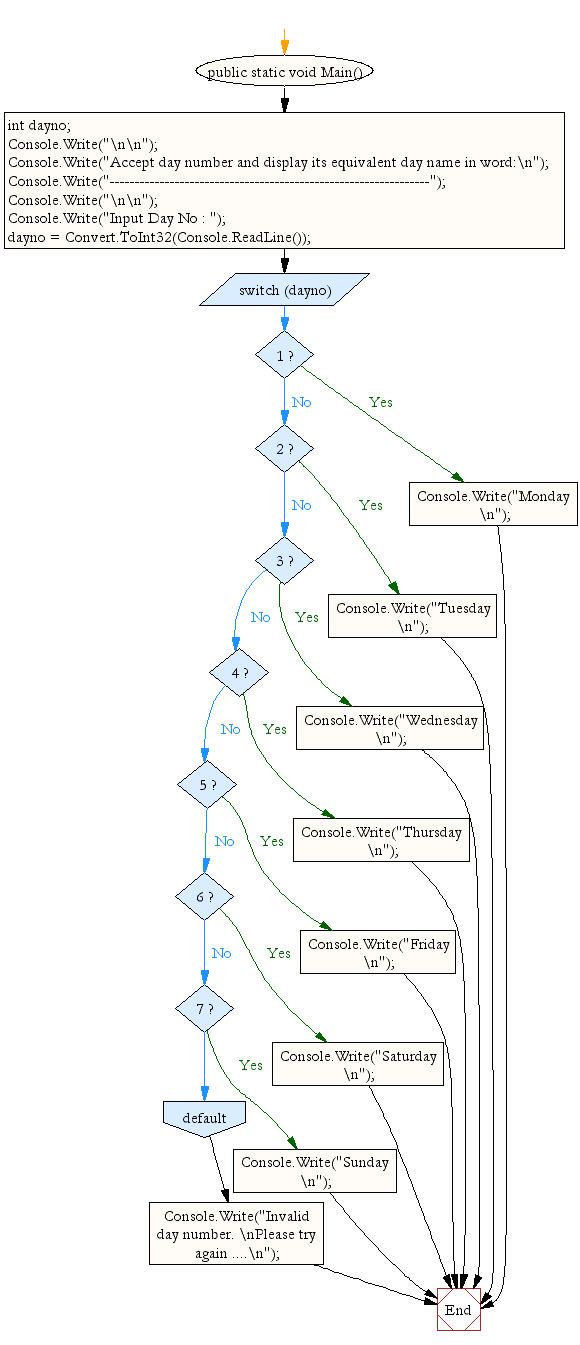
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C# Sharp to accept a grade and display the equivalent description.
Next: Write a program in C# Sharp to read any digit, display in the word.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics