C#: Accept a grade and display equivalent description
Write a program in C# Sharp to accept a grade and display the equivalent description:
Grade | Description |
---|---|
E | Excellent |
V | Very Good |
G | Good |
A | Average |
F | Fail |
Sample Solution:-
C# Sharp Code:
using System; // Importing necessary namespaces
public class exercise19 // Declaration of the exercise19 class
{
static void Main(string[] args) // Entry point of the program
{
string notes; // Declaration of a variable to store grade description
char grd; // Declaration of a variable to store the grade as a character
Console.Write("\n\n"); // Printing new lines
Console.Write("Accept a grade and display equivalent description:\n"); // Displaying the purpose of the program
Console.Write("---------------------------------------------------"); // Displaying a separator
Console.Write("\n\n");
Console.Write("Input the grade :"); // Prompting user to input a grade
grd = Convert.ToChar(Console.ReadLine().ToUpper()); // Reading the input and converting it to uppercase
// Using a switch statement to match the grade and assign a description
switch(grd)
{
case 'E':
notes = " Excellent"; // Assigning "Excellent" for grade 'E'
break;
case 'V':
notes = " Very Good"; // Assigning "Very Good" for grade 'V'
break;
case 'G':
notes = " Good "; // Assigning "Good" for grade 'G'
break;
case 'A':
notes = " Average"; // Assigning "Average" for grade 'A'
break;
case 'F':
notes = " Fails"; // Assigning "Fails" for grade 'F'
break;
default :
notes = "Invalid Grade Found."; // Handling default case for invalid grades
break;
}
Console.Write("You have chosen : {0}\n", notes); // Printing the chosen grade and its description
}
}
Sample Output:
Accept a grade and display equivalent description: --------------------------------------------------- Input the grade :V You have chosen : Very Good
Visual Presentation:
Flowchart:
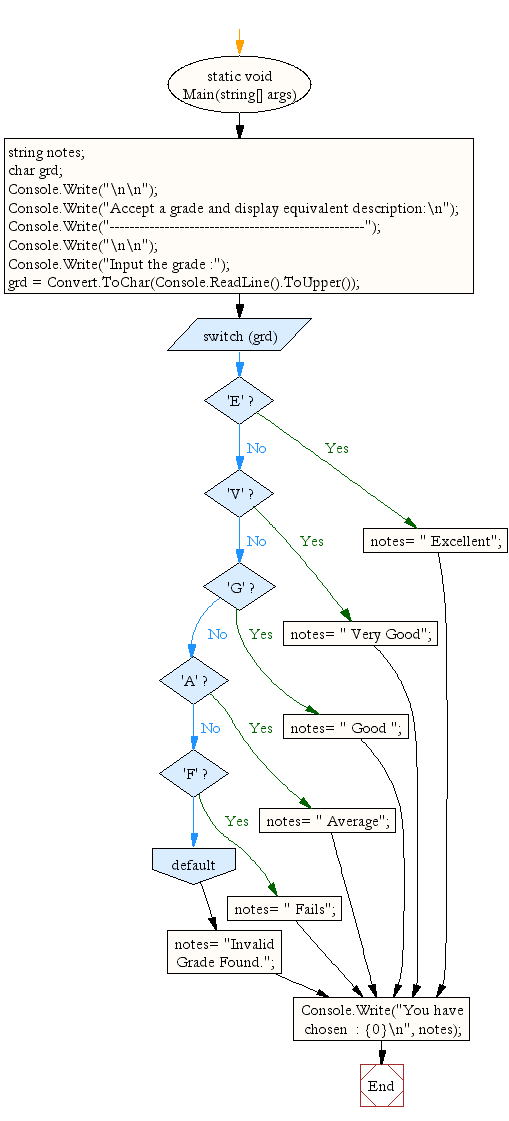
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C# Sharp to calculate and print the Electricity bill of a given customer.
Next: Write a program in C# Sharp to read any day number in integer and display day name in the word.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.