C#: Calculate profit and loss
Write a C# Sharp program to calculate profit and loss on a transaction.
Sample Solution:-
C# Sharp Code:
using System; // Importing the System namespace
public class Exercise17 // Declaration of the Exercise17 class
{
public static void Main() // Entry point of the program
{
int cprice, sprice, plamt; // Declaration of variables to store cost price, selling price, and profit/loss amount
Console.Write("\n\n"); // Printing new lines
Console.Write("Calculate profit and loss:\n"); // Displaying the purpose of the program
Console.Write("----------------------------"); // Displaying a separator
Console.Write("\n\n");
Console.Write("Input Cost Price: "); // Prompting user to input the cost price
cprice = Convert.ToInt32(Console.ReadLine()); // Reading the input and converting it to an integer
Console.Write("Input Selling Price: "); // Prompting user to input the selling price
sprice = Convert.ToInt32(Console.ReadLine()); // Reading the input and converting it to an integer
if (sprice > cprice) // Checking if selling price is greater than cost price
{
plamt = sprice - cprice; // Calculating profit amount
Console.Write("\nYou can book your profit amount : {0}\n", plamt); // Printing the profit amount
}
else if (cprice > sprice) // Checking if cost price is greater than selling price
{
plamt = cprice - sprice; // Calculating loss amount
Console.Write("\nYou got a loss of amount : {0}\n", plamt); // Printing the loss amount
}
else // Handling scenario where cost price equals selling price
{
Console.Write("\nYou are running in no profit no loss condition.\n"); // Printing a message for no profit and no loss
}
}
}
Sample Output:
Calculate profit and loss: ---------------------------- Input Cost Price: 1000 Input Selling Price: 1200 You can booked your profit amount : 200
Visual Presentation:
Pictorial Presentation:
Flowchart:
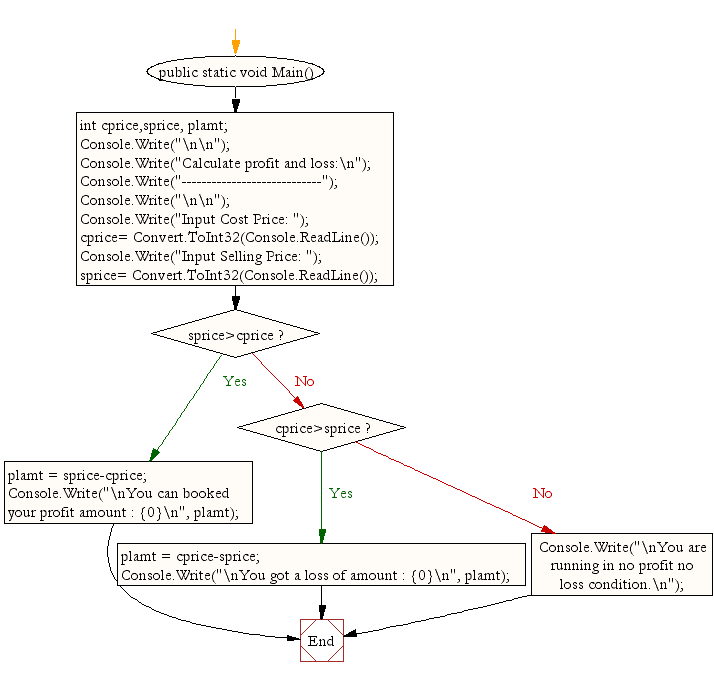
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C# Sharp program to check whether an alphabet is a vowel or consonant.
Next: Write a program in C# Sharp to calculate and print the Electricity bill of a given customer.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.