C#: Check whether an alphabet is a vowel or consonant
C# Sharp Conditional Statement: Exercise-16 with Solution
Write a C# Sharp program to check whether an alphabet letter is a vowel or a consonant.
Sample Solution:-
C# Sharp Code:
using System; // Importing necessary namespaces
public class exercise16 // Declaration of the exercise16 class
{
static void Main(string[] args) // Entry point of the program
{
char ch; // Declaration of a character variable to store the input
Console.Write("\n\n"); // Printing new lines
Console.Write("check whether the input alphabet is a vowel or not:\n"); // Displaying the purpose of the program
Console.Write("-----------------------------------------------------"); // Displaying a separator
Console.Write("\n\n");
Console.Write("Input an Alphabet (A-Z or a-z) : "); // Prompting user to input an alphabet
ch = Convert.ToChar(Console.ReadLine().ToLower()); // Reading the input and converting it to lowercase
int i = ch; // Converting the character to its corresponding ASCII value
if (i >= 48 && i <= 57) // Checking if the input is a number
{
Console.Write("You entered a number, Please enter an alphabet."); // Prompting user to enter an alphabet
}
else
{
switch (ch) // Switch statement to check for vowels
{
case 'a':
Console.WriteLine("The Alphabet is vowel"); // Printing a message if 'a' is entered
break;
case 'i':
Console.WriteLine("The Alphabet is vowel"); // Printing a message if 'i' is entered
break;
case 'o':
Console.WriteLine("The Alphabet is vowel"); // Printing a message if 'o' is entered
break;
case 'u':
Console.WriteLine("The Alphabet is vowel"); // Printing a message if 'u' is entered
break;
case 'e':
Console.WriteLine("The Alphabet is vowel"); // Printing a message if 'e' is entered
break;
default:
Console.WriteLine("The Alphabet is not a vowel"); // Printing a message for non-vowel characters
break;
}
}
Console.ReadKey(); // Waiting for a key press before closing the console window
}
}
Sample Output:
check whether the input alphabet is a vowel or not: ----------------------------------------------------- Input an Alphabet : A The Alphabet is vowel
Visual Presentation:
Flowchart:
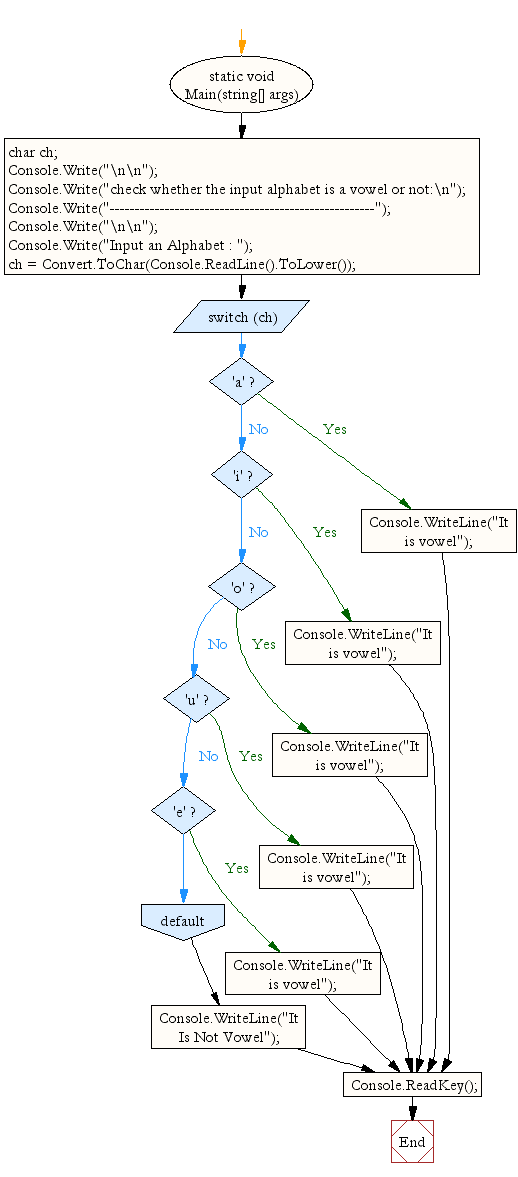
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C# Sharp program to check whether a triangle can be formed by the given value for the angles.
Next: Write a C# Sharp program to calculate profit and loss on a transaction.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/csharp-exercises/conditional-statement/csharp-conditional-statement-exercise-16.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics