C#: String with same characters
C# Sharp Basic: Exercise-96 with Solution
Write a C# Sharp program to check whether all characters in a string are the same. Return true if all the characters in the string are the same, otherwise false.
Sample Data:
("aaa") -> True
("abcd") -> False
("3333") -> True
("2342342") -> False
Sample Solution:
C# Sharp Code:
using System;
namespace exercises
{
class Program
{
static void Main(string[] args)
{
// Initializing different strings
string text = "aaa";
Console.WriteLine("Original string: " + text);
Console.WriteLine("Check whether all the characters in the said string are same or not! " + test(text));
text = "abcd";
Console.WriteLine("Original string: " + text);
Console.WriteLine("Check whether all the characters in the said string are same or not! " + test(text));
text = "3333";
Console.WriteLine("Original string: " + text);
Console.WriteLine("Check whether all the characters in the said string are same or not! " + test(text));
text = "2342342";
Console.WriteLine("Original string: " + text);
Console.WriteLine("Check whether all the characters in the said string are same or not! " + test(text));
}
// Method to check if all characters in a string are the same
public static bool test(string text)
{
// Check if the length of the string is greater than 1
if (text.Length > 1)
{
var b = text[0]; // Store the first character of the string
// Loop through the characters starting from the second character
for (int i = 1; i < text.Length; i++)
{
var c = text[i]; // Store the current character
// If the current character is not equal to the first character, return false
if (c != b)
{
return false;
}
}
}
return true; // Return true if all characters are the same or if the string has only one character
}
}
}
Sample Output:
Original string: aaa Check whether all the characters in the said string are same or not! True Original string: abcd Check whether all the characters in the said string are same or not! False Original string: 3333 Check whether all the characters in the said string are same or not! True Original string: 2342342 Check whether all the characters in the said string are same or not! False
Flowchart:
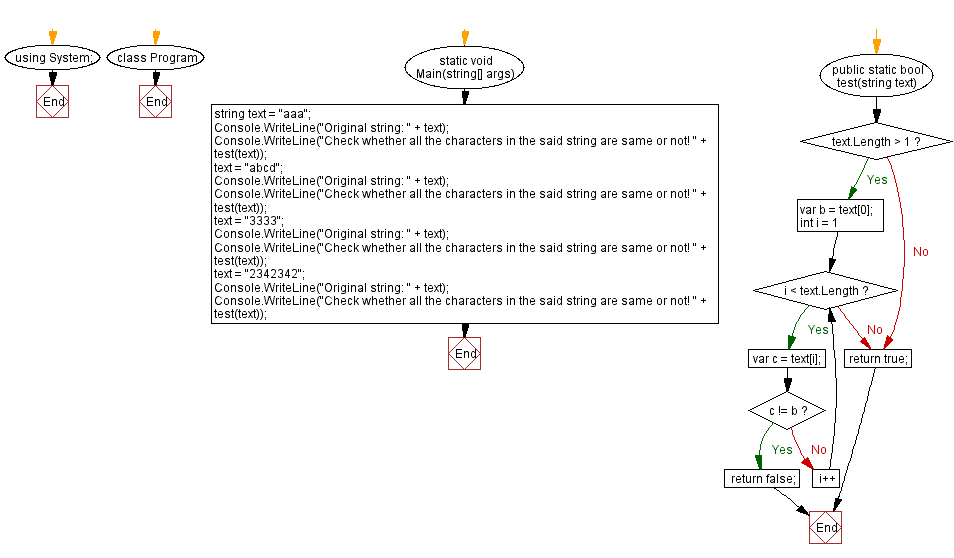
C# Sharp Code Editor:
Previous C# Sharp Exercise: Verify that a string contains valid parentheses.
Next C# Sharp Exercise: Check if a string is numeric or not.
What is the difficulty level of this exercise?
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/csharp-exercises/basic/csharp-basic-exercise-96.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics