C#: Square root of a given number
C# Sharp Basic: Exercise-93 with Solution
Write a C# Sharp program to calculate the square root of a given number. Return the integer part of the result instead of using any built-in functions.
Sample Data:
(120) -> 10
(225) -> 15
(335) -> 18
Sample Solution:
C# Sharp Code:
using System;
namespace exercises
{
class Program
{
static void Main(string[] args)
{
// Initializing integer 'n' with various values
int n = 120;
Console.WriteLine("Original number: " + n);
// Displaying the square root of 'n'
Console.WriteLine("Square root of the said number: " + test(n));
// Changing the value of 'n' and repeating the process
n = 225;
Console.WriteLine("\nOriginal number: " + n);
Console.WriteLine("Square root of the said number: " + test(n));
// Changing the value of 'n' and repeating the process
n = 335;
Console.WriteLine("\nOriginal number: " + n);
Console.WriteLine("Square root of the said number: " + test(n));
}
// Method to calculate the square root of a given number 'n'
public static int test(double n)
{
int sq = 1;
// Loop to find the square root using an iterative approach
while (sq < n / sq)
{
sq++;
}
// Checking if the square is greater than 'n/square'. If so, returns the square - 1
if (sq > n / sq)
return sq - 1;
return sq; // Returning the square root of 'n'
}
}
}
Sample Output:
Original number: 120 Square root of the said number: 10 Original number: 225 Square root of the said number: 15 Original number: 335 Square root of the said number: 18
Flowchart:
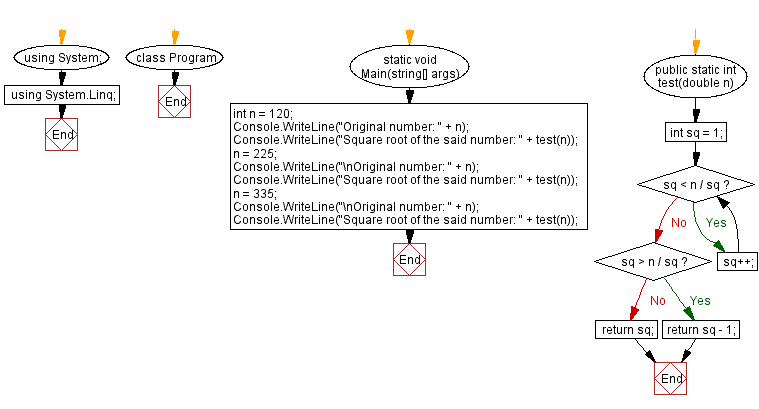
C# Sharp Code Editor:
Previous C# Sharp Exercise: Next prime number of a given integer.
Next C# Sharp Exercise: Find the longest common prefix from an array of strings.
What is the difficulty level of this exercise?
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/csharp-exercises/basic/csharp-basic-exercise-93.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics