C#: Remove all the values except integer values from a given array of mixed values
Remove Non-Integer Values from Array
Write a C# Sharp program to remove all values except integer values from a given array of mixed values.
Sample Solution:
C# Sharp Code:
using System;
using System.Linq;
namespace exercises
{
class Program
{
static void Main(string[] args)
{
// Initializing an array of objects with various types of values
object[] mixedArray = new object[6];
mixedArray[0] = 25;
mixedArray[1] = "Anna";
mixedArray[2] = false;
mixedArray[3] = System.DateTime.Now;
mixedArray[4] = -112;
mixedArray[5] = -34.67;
// Displaying the original elements of the mixed array
Console.WriteLine("Original array elements:");
for (int i = 0; i < mixedArray.Length; i++)
{
Console.Write(mixedArray[i] + " ");
}
// Calling the 'test' method to extract integer values from the mixed array
int[] new_nums = test(mixedArray);
// Displaying the integer values extracted from the mixed array
Console.WriteLine("\n\nAfter removing all the values except integer values from the said array of mixed values:");
for (int i = 0; i < new_nums.Length; i++)
{
Console.Write(new_nums[i] + " ");
}
}
// Method to extract integer values from an array of objects
public static int[] test(object[] nums)
{
// Using LINQ's 'OfType' to filter and convert objects to integers and returning as an array
return nums.OfType<int>().ToArray();
}
}
}
Sample Output:
Original array elements: 25 Anna False 4/24/2021 11:43:11 AM -112 -34.67 After removing all the values except integer values from the said array of mixed values: 25 -112
Flowchart:
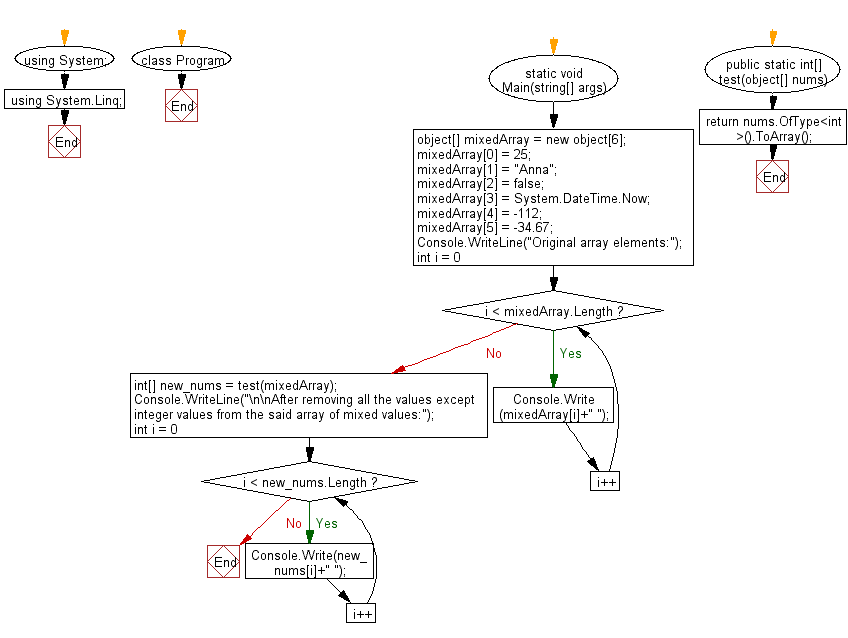
For more Practice: Solve these Related Problems:
- Write a C# program to extract only negative integers from a mixed-type array and return them in reverse order.
- Write a C# program to remove all elements except even integers from a heterogeneous list.
- Write a C# program to filter and sum all integer values in an array containing strings, floats, and booleans.
- Write a C# program to separate integers from a mixed array and sort them in descending order.
Go to:
PREV : Count Ones and Zeros in Binary.
NEXT : Find Next Prime Number.
C# Sharp Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.