C#: Count positive and negative numbers in a given array of integers
C# Sharp Basic: Exercise-89 with Solution
Write a C# Sharp program to count positive and negative numbers in a given array of integers.
Sample Solution:
C# Sharp Code:
using System;
using System.Linq;
namespace exercises
{
class Program
{
static void Main(string[] args)
{
// Array declaration and initialization with integer elements
int[] nums = { 10, -11, 12, -13, 14, -18, 19, -20 };
// Displaying the original elements of the array 'nums'
Console.WriteLine("Original Array elements:");
foreach (var item in nums)
{
Console.Write(item.ToString() + " ");
}
// Calling the 'test' method and displaying the count of positive and negative numbers in the array 'nums'
Console.WriteLine(test(nums));
// Another array declaration and initialization with integer elements
int[] nums1 = { -4, -3, -2, 0, 3, 5, 6, 2, 6 };
// Displaying the original elements of the array 'nums1'
Console.WriteLine("\nOriginal Array elements:");
foreach (var item in nums1)
{
Console.Write(item.ToString() + " ");
}
// Calling the 'test' method and displaying the count of positive and negative numbers in the array 'nums1'
Console.WriteLine(test(nums1));
// Empty array declaration (no elements)
int[] nums2 = {};
// Displaying the original elements of the array 'nums2' (empty array)
Console.WriteLine("\nOriginal Array elements:");
foreach (var item in nums2)
{
Console.Write(item.ToString() + " ");
}
// Calling the 'test' method for the empty array 'nums2' and displaying the count of positive and negative numbers
Console.WriteLine(test(nums2));
}
// Method to count the number of positive and negative numbers in an integer array
public static string test(int[] nums)
{
// Filtering positive and negative numbers using LINQ 'Where' clause
var pos = nums.Where(n => n > 0);
var neg = nums.Where(n => n < 0);
// Returning the count of positive and negative numbers as a formatted string
return "\nNumber of positive numbers: " + pos.Count() + "\nNumber of negative numbers: " + neg.Count();
}
}
}
Sample Output:
Original Array elements: 10 -11 12 -13 14 -18 19 -20 Number of positive numbers: 4 Number of negative numbers: 4 Original Array elements: -4 -3 -2 0 3 5 6 2 6 Number of positive numbers: 5 Number of negative numbers: 3 Original Array elements: Number of positive numbers: 0 Number of negative numbers: 0
Flowchart:
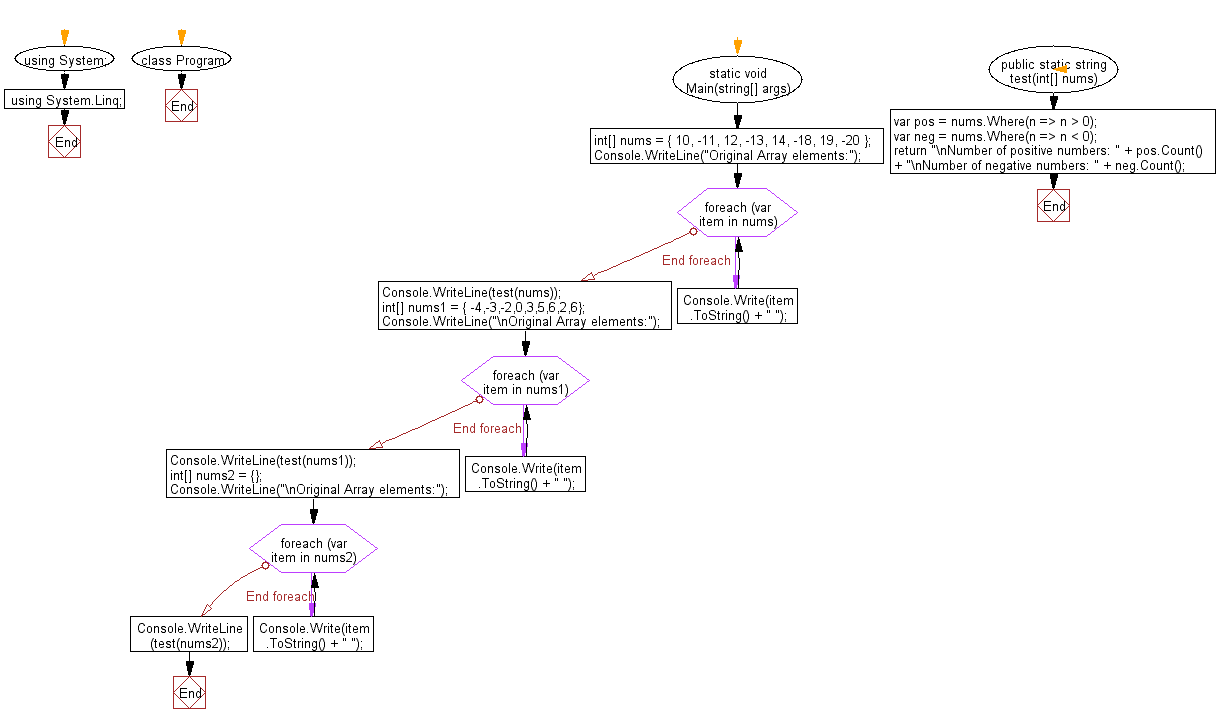
C# Sharp Code Editor:
Previous: Write a C# Sharp program to find the sum of the interior angles (in degrees) of a given Polygon. Input number of straight line(s).
Next: Write a C# Sharp program to count number of ones and zeros in the binary representation of a given integer.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/csharp-exercises/basic/csharp-basic-exercise-89.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics