C# Sharp Exercises: Find the sum of the interior angles (in degrees) of a given Polygon
Sum of Interior Angles in Polygon
Write a C# Sharp program to find the sum of the interior angles (in degrees) of a given polygon. Input the number of straight lines.
From Wikipedia,
In geometry, a polygon is a plane figure that is described by a finite number of straight line segments connected to form a closed polygonal chain or polygonal circuit. The solid plane region, the bounding circuit, or the two together, may be called a Polygon.
Sample Solution:
C# Sharp Code:
using System;
namespace exercises
{
class Program
{
static void Main(string[] args)
{
// Asking the user to input the number of straight lines in the polygon
Console.WriteLine("Input number of straight lines of the polygon:");
// Reading the input number from the user and converting it to an integer
int n = Convert.ToInt32(Console.ReadLine());
// Displaying the sum of the interior angles of the polygon using the 'test' method
Console.WriteLine("Sum of the interior angles (in degrees) of the said polygon: " + test(n));
}
// Method to calculate the sum of interior angles of a polygon
public static int test(int num)
{
// Formula to calculate the sum of interior angles of a polygon
return 180 * (num - 2);
}
}
}
Sample Output:
Input number of straight lines of the polygon: Sum of the interior angles (in degrees) of the said polygon: -360
Flowchart:
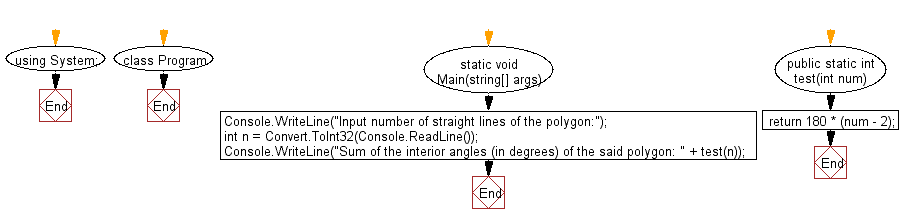
For more Practice: Solve these Related Problems:
- Write a C# program to calculate the sum of exterior angles for a given polygon with 'n' sides.
- Write a C# program to validate if a polygon with given side lengths can exist and return its interior angle sum.
- Write a C# program to return the average interior angle of a polygon based on number of sides.
- Write a C# program to compare the sum of interior angles of two different polygons and return the difference.
Go to:
PREV : Reverse Boolean Value.
NEXT : Count Positives and Negatives in Array.
C# Sharp Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.