C#: Reverse a boolean value
Reverse Boolean Value
Write a C# Sharp program to reverse a Boolean value.
Sample Solution:
C# Sharp Code:
using System;
namespace exercises
{
class Program
{
static void Main(string[] args)
{
// Declaring and initializing boolean variables
bool cat = false;
bool dog = true;
// Displaying the original value of the 'cat' variable
Console.WriteLine("Original value: " + cat);
// Displaying the reversed value of 'cat' using the 'test' method
Console.WriteLine("Reverse value: " + test(cat));
// Displaying the original value of the 'dog' variable
Console.WriteLine("Original value: " + dog);
// Displaying the reversed value of 'dog' using the 'test' method
Console.WriteLine("Reverse value: " + test(dog));
}
// Method to reverse a boolean value
public static bool test(bool boolean)
{
// Reversing the boolean value using the logical NOT operator (!)
return !boolean;
}
}
}
Sample Output:
Original value: False Reverse value: True Original value: True Reverse value: False
Flowchart:
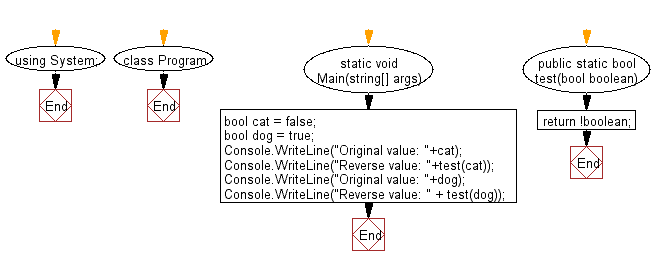
For more Practice: Solve these Related Problems:
- Write a C# program to toggle a Boolean value only if it's part of a conditionally evaluated expression.
- Write a C# program to evaluate the reverse of Boolean values inside a nested dictionary structure.
- Write a C# program to reverse an array of Boolean values and return the result in reversed order.
- Write a C# program to flip a Boolean value if it occurs an even number of times in an array.
Go to:
PREV : Count Letters and Digits in String.
NEXT : Sum of Interior Angles in Polygon.
C# Sharp Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.