C#: Index number of all lower case letters in a given string
Indices of Lowercase Letters
Write a C# Sharp program to get the index number of all lower case letters in a given string.
Sample Solution:
C# Sharp Code:
using System;
using System.Linq;
using System.Collections.Generic;
namespace exercises
{
class Program
{
static void Main(string[] args)
{
// Declaring a string variable
string text;
// Testing the function test with different input strings
// String containing only letters (Python)
text = "Python";
Console.WriteLine("Orginal string: " + text);
// Calling the test method to get the indices of lowercase letters in the string
int[] result = test(text);
// Displaying the indices of lowercase letters in the string
Console.WriteLine("\nIndices of all lower case letters of the said string:");
foreach (var item in result)
{
Console.Write(item.ToString() + " ");
}
// Another string containing only letters (JavaScript)
text = "JavaScript";
Console.WriteLine("\nOrginal string: " + text);
// Calling the test method to get the indices of lowercase letters in this string
result = test(text);
// Displaying the indices of lowercase letters in this string
Console.WriteLine("\nIndices of all lower case letters of the said string:");
foreach (var item in result)
{
Console.Write(item.ToString() + " ");
}
}
// Method to find indices of lowercase letters in a string
public static int[] test(string str)
{
// Using LINQ to select indices where the characters are lowercase in the string
return str.Select((x, i) => i).Where(i => char.IsLower(str[i])).ToArray();
}
}
}
Sample Output:
Orginal string: Python Indices of all lower case letters of the said string: 1 2 3 4 5 Orginal string: JavaScript Indices of all lower case letters of the said string: 1 2 3 5 6 7 8 9
Flowchart:
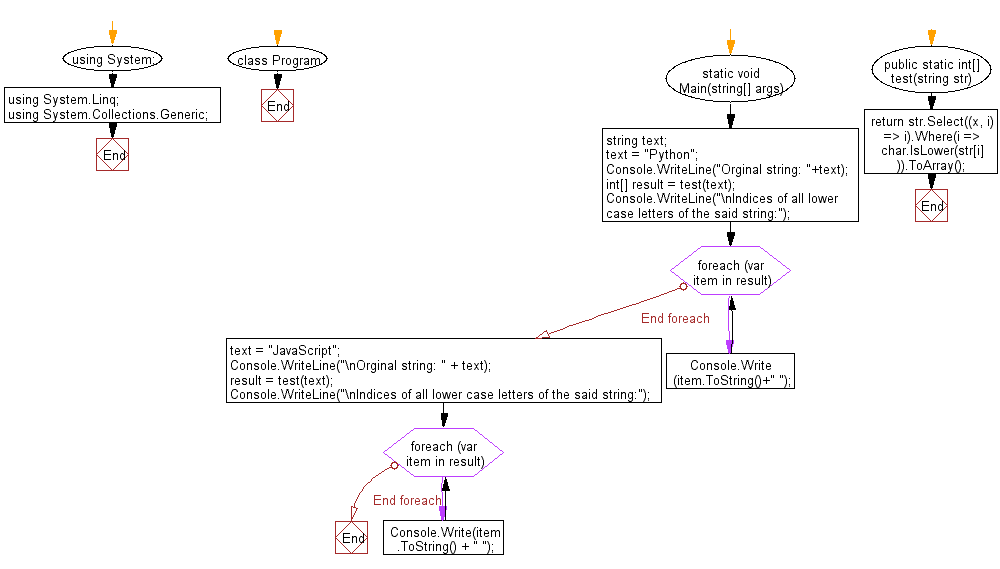
C# Sharp Code Editor:
Previous:Write a C# Sharp program to remove all vowels from a given string.
Next: Write a C# Sharp program to find the cumulative sum of an array of number.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics