C#: Remove all characters which are non-letters from a given string
Remove Non-Letter Characters
Write a C# Sharp program to remove all non-letter characters from a given string.
From Wikipedia,
A letter is a segmental symbol of a phonemic writing system. The inventory of all letters forms the alphabet. Letters broadly correspond to phonemes in the spoken form of the language, although there is rarely a consistent, exact correspondence between letters and phonemes
Sample Solution:
C# Sharp Code:
using System;
using System.Text.RegularExpressions;
namespace exercises
{
class Program
{
static void Main(string[] args)
{
// Declaring a string variable
string text;
// Testing the function test with different input strings
// String containing letters, numbers, and special characters
text = "Py@th12on";
Console.WriteLine("Orginal string: " + text);
Console.WriteLine("Remove all characters from the said string which are non-letters: " + test(text));
// String containing letters, numbers, and spaces
text = "Python 3.0";
Console.WriteLine("\nOrginal string: " + text);
Console.WriteLine("Remove all characters from the said string which are non-letters: " + test(text));
// String containing letters, numbers, and special characters
text = "2^sdfds*^*^jlljdslfnoswje34u230sdfds984";
Console.WriteLine("\nOrginal string: " + text);
Console.WriteLine("Remove all characters from the said string which are non-letters: " + test(text));
}
// Method to remove non-letter characters from a string using Regex
public static string test(string text)
{
// Using Regex.Replace to remove characters that are not letters (a-zA-Z)
return Regex.Replace(text, @"[^a-zA-Z]", "");
}
}
}
Sample Output:
Orginal string: Py@th12on Remove all characters from the said string which are non-letters: Python Orginal string: Python 3.0 Remove all characters from the said string which are non-letters: Python Orginal string: 2^sdfds*^*^jlljdslfnoswje34u230sdfds984 Remove all characters from the said string which are non-letters: sdfdsjlljdslfnoswjeusdfds
Flowchart:
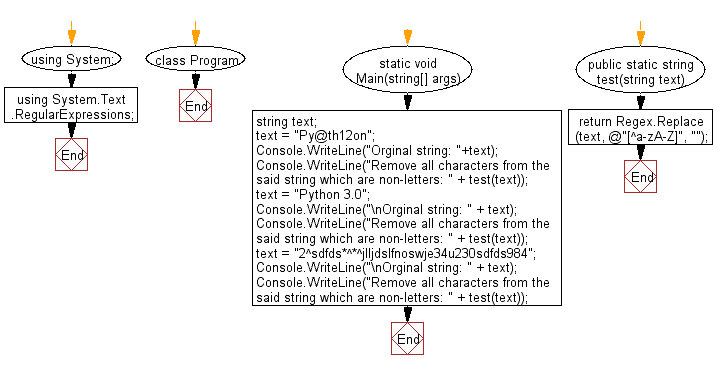
C# Sharp Code Editor:
Previous:Write a C# Sharp program to swap a two digit given number and check whether the given number is greater than its swap value.
Next:Write a C# Sharp program to remove all vowels from a given string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics