C#: Get the ASCII value of a given character
Get ASCII Value of Character
Write a C# Sharp program to get the ASCII value of a given character.
Sample Solution:
C# Sharp Code:
using System;
using System.Linq;
namespace exercises
{
class Program
{
// Main method where the program execution begins
static void Main(string[] args)
{
// Display the ASCII values of different characters
Console.WriteLine("Ascii value of 1 is: " + test('1'));
Console.WriteLine("Ascii value of A is: " + test('A'));
Console.WriteLine("Ascii value of a is: " + test('a'));
Console.WriteLine("Ascii value of # is: " + test('#'));
}
// Function to obtain the ASCII value of a character
public static int test(char ch)
{
return (int)ch; // Casting the character to an integer gives its ASCII value
}
}
}
Sample Output:
Ascii value of 1 is: 49 Ascii value of A is: 65 Ascii value of a is: 97 Ascii value of # is: 35
Flowchart:
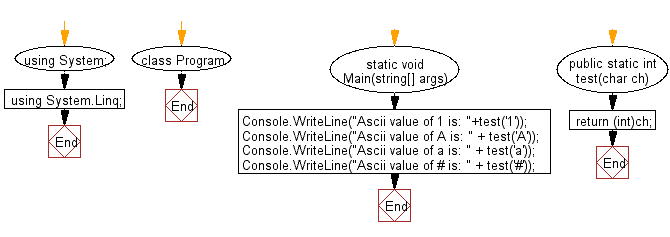
For more Practice: Solve these Related Problems:
- Write a C# program to print the ASCII value of each character in a string and identify the highest and lowest ASCII values.
- Write a C# program to convert a string to its ASCII byte array and reconstruct the string from that array.
- Write a C# program to find the sum of ASCII values of all characters in a user-input sentence.
- Write a C# program to determine whether a character is printable based on its ASCII value.
Go to:
PREV : Nth Odd Number.
NEXT : Check Word Plural.
C# Sharp Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.