C#: Take a positive number and return the nth odd number
Nth Odd Number
Write a C# Sharp program that takes a positive number and returns the nth odd number.
Sample Solution:
C# Sharp Code:
using System;
using System.Linq;
namespace exercises
{
class Program
{
// Main method where the program execution begins
static void Main(string[] args)
{
// Display the odd numbers at different positions
Console.WriteLine("1st odd number: " + test(1));
Console.WriteLine("2nd odd number: " + test(2));
Console.WriteLine("4th odd number: " + test(4));
Console.WriteLine("100th odd number: " + test(100));
}
// Function to calculate the nth odd number
public static int test(int n)
{
return n * 2 - 1; // The nth odd number can be calculated using this formula
}
}
}
Sample Output:
1st odd number: 1 2nd odd number: 3 4th odd number: 7 100th odd number: 199
Flowchart:
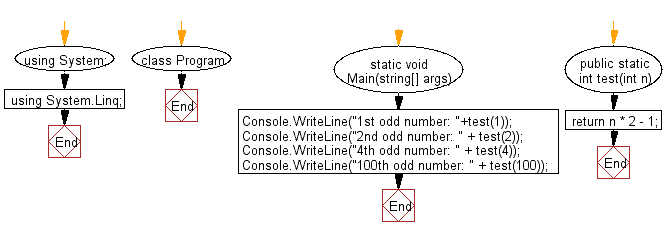
C# Sharp Code Editor:
Previous: Write a C# Sharp program to check the length of a given string is odd or even. Return 'Odd length' if the string length is odd otherwise 'Even length'.
Next: Write a C# Sharp program to get the ASCII value of a given character.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.