C#: Sort the integers in ascending order without moving the number -5
Sort Integers Keeping -5 Fixed
Write a C# program to sort the integers in ascending order without moving the number -5.
Sample Solution:
C# Sharp Code:
using System;
using System.Linq;
public class Example
{
// Function to sort numbers in an array, keeping -5 values in their original positions
public static int[] sort_numbers(int[] arra)
{
// Extract and sort non-negative numbers (excluding -5)
int[] num = arra.Where(x => x != -5).OrderBy(x => x).ToArray();
int ctr = 0; // Counter for non-negative numbers used in sorting
// Map sorted non-negative numbers back to the array while preserving -5 values
return arra.Select(x => x >= 0 ? num[ctr++] : -5).ToArray();
}
// Main method to test the sort_numbers function
public static void Main()
{
// Test the sort_numbers function with an array and print the result
int[] x = sort_numbers(new int[] {-5, 236, 120, 70, -5, -5, 698, 280 });
// Print each element of the resulting array
foreach(var item in x)
{
Console.WriteLine(item.ToString());
}
}
}
Sample Output:
-5 70 120 236 -5 -5 280 698
Flowchart:
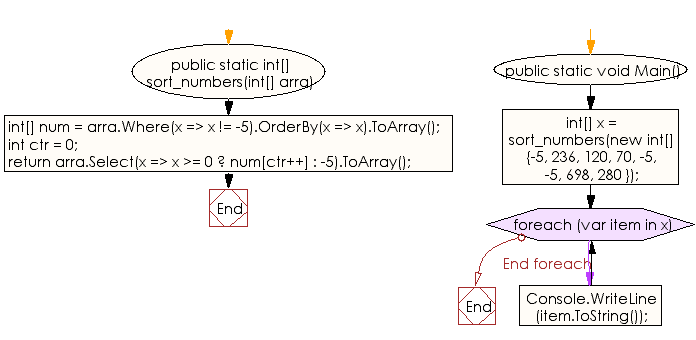
For more Practice: Solve these Related Problems:
- Write a C# program to sort all positive numbers in an array without moving any negative number.
- Write a C# program to sort an array while keeping every instance of the number 0 in the same place.
- Write a C# program to sort all numbers except those divisible by 5, which must remain in place.
- Write a C# program to sort all elements except the maximum and minimum values.
Go to:
PREV : Sum of Matrix with Zero Condition.
NEXT : Reverse Strings in Parentheses.
C# Sharp Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.