C#: Checks how many integers are needed to complete the range
Complete Missing Numbers in Range
Write a C# program that accepts a list of integers and checks how many integers are needed to complete the range.
For example [1, 3, 4, 7, 9], between 1-9 -> 2, 5, 6, 8 are not present in the list. So output will be 4.
Sample Solution:
C# Sharp Code:
using System;
public class Example
{
// Function to count the number of elements required to make an array consecutive
public static int consecutive_array(int[] input_Array)
{
// Sorting the input array in ascending order
Array.Sort(input_Array);
// Counter variable to track the number of elements needed to make the array consecutive
int ctr = 0;
// Loop through the sorted array to calculate the number of elements needed to fill the gaps
for (int i = 0; i < input_Array.Length - 1; i++)
{
// Increment the counter by the difference between adjacent elements minus 1
// This calculates the number of missing elements between consecutive elements
ctr += input_Array[i + 1] - input_Array[i] - 1;
}
// Return the count of elements needed to make the array consecutive
return ctr;
}
// Main method to test the consecutive_array function
public static void Main()
{
// Testing the consecutive_array function with different input arrays
Console.WriteLine(consecutive_array(new int[] {1, 3, 5, 6, 9})); // Output: 2
Console.WriteLine(consecutive_array(new int[] {0, 10})); // Output: 8
}
}
Sample Output:
4 9
Flowchart:
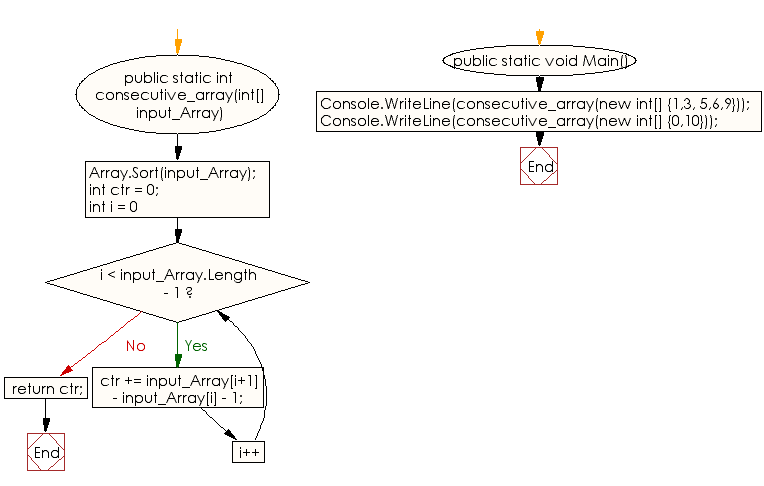
C# Sharp Code Editor:
Previous: Write a C# program to find the pair of adjacent elements that has the highest product of an given array of integers.
Next: Write a C# program to check whether it is possible to create a strictly increasing sequence from a given sequence of integers as an array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics