C#: Find the pair of adjacent elements that has the highest product of an given array of integers
Max Product of Adjacent Integers
Write a C# program to find the pair of adjacent elements that has the highest product of an array of integers.
Sample Solution:
C# Sharp Code:
Sample Output:
-3 20 10 0
Flowchart:
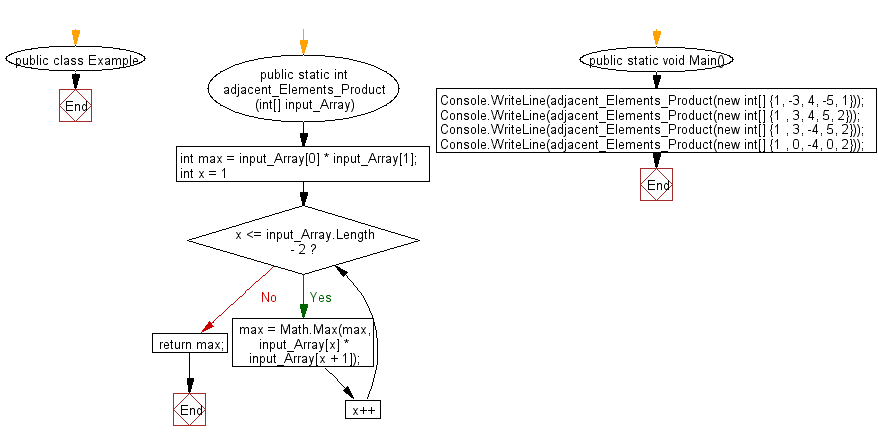
C# Sharp Code Editor:
Previous: Write a C# program to check if a given string is a palindrome or not.
Next: Write a C# program which will accept a list of integers and checks how many integers are needed to complete the range.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.