C#: Count a specified number in a given array of integers
Count Specific Number in Array
Write a C# program to count a specified number in a given array of integers.
Pictorial Presentation:
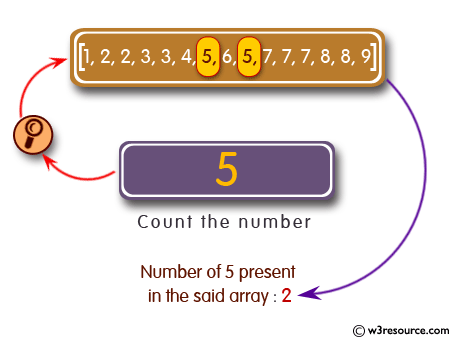
Sample Solution:
C# Sharp Code:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
public class Exercise45 {
static void Main(string[] args) {
// Prompt the user to input an integer
Console.WriteLine("\nInput an integer:");
// Read the input integer and store it in the variable 'x'
int x = Convert.ToInt32(Console.ReadLine());
// Define an array of integers 'nums' with pre-defined values
int[] nums = {1, 2, 2, 3, 3, 4, 5, 6, 5, 7, 7, 7, 8, 8, 9};
// Display a message indicating the number being searched for in the array
Console.WriteLine("Number of " + x + " present in the said array:");
// Count the occurrences of the input integer 'x' in the 'nums' array and print the count
Console.WriteLine(nums.Count(n => n == x));
}
}
Sample Output:
Input an integer: 5 Number of 5 present in the said array: 2
Flowchart:
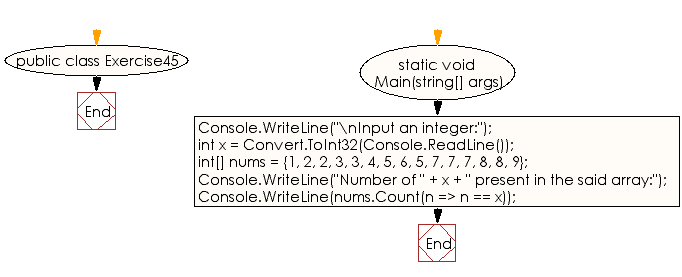
C# Sharp Code Editor:
Previous: Write a C# program to create a new string of every other character (odd position) from the first position of a given string.
Next: Write a C# program to check if a number appears as either the first or last element of an array of integers and the length is 1 or more.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.