C#: Create a new string of every other character from the first position of a given string
Every Other Character in String
Write a C# program to create a string of every other character (odd position) from the first position of a given string.
Pictorial Presentation:
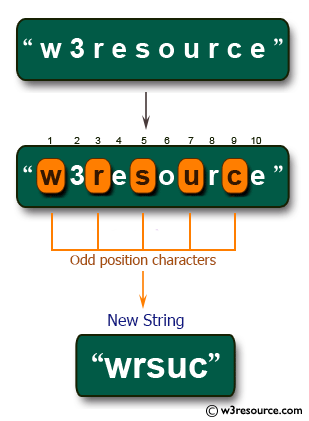
Sample Solution:-
C# Sharp Code:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
public class Exercise44 {
static void Main(string[] args) {
// Prompt the user to input a string
Console.Write("Input a string : ");
// Read the input string and store it in the variable 'str'
string str = Console.ReadLine();
// Initialize an empty string variable 'result' to store the characters at even indices
var result = string.Empty;
// Iterate through the characters of the input string using a for loop
for (var i = 0; i < str.Length; i++) {
// Check if the index 'i' is even (i.e., divisible by 2 without remainder)
// If the index is even, add the character at index 'i' to the 'result' string
if (i % 2 == 0)
result += str[i];
}
// Output the 'result' string containing characters at even indices from the input string
Console.WriteLine(result);
}
}
Sample Output:
Input a string : w3resource wrsuc
Flowchart:
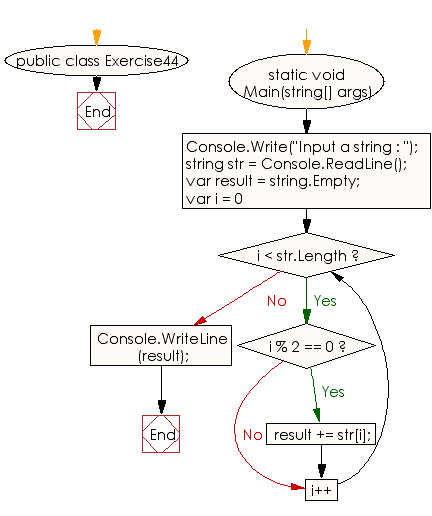
C# Sharp Code Editor:
Previous: Write a C# program to check if a given string starts with "w" and immediately followed by two "ww".
Next: Write a C# program to count a specified number in a given array of integers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.