C#: Check if a given string starts with "w" and immediately followed by two "ww"
Check String Starts with 'www'
Write a C# program to check if a given string starts with "w" and is immediately followed by two "ww".
Sample Solution:
C# Sharp Code:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
public class Exercise43 {
static void Main(string[] args) {
// Prompt the user to input a string
Console.Write("Input a string : ");
// Read the input string and store it in the variable 'str'
string str = Console.ReadLine();
// Call the 'test' method with the input string and output the result
Console.WriteLine(test(str));
}
// Define a method named 'test' that takes a string parameter 'str' and returns a boolean value
public static bool test(string str) {
var ctr = 0; // Initialize a counter variable 'ctr' to count occurrences of 'w'
// Iterate through the characters of the string using a for loop
for (var i = 0; i < str.Length - 1; i++) {
// Check if the current character is 'w'; if so, increment the counter 'ctr'
if (str[i].Equals('w'))
ctr++;
// Check if the substring of length 2 starting at index 'i' contains "ww"
// and if the counter 'ctr' is greater than 2
if (str.Substring(i, 2).Equals("ww") && ctr > 2)
return true; // If the condition is met, return 'true'
}
return false; // Return 'false' if the condition is not met throughout the string
}
}
Sample Output:
Input a string : www False
Flowchart:
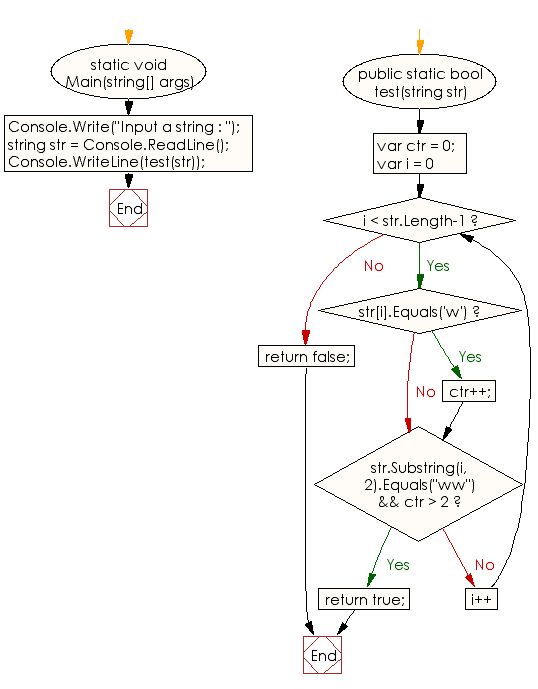
C# Sharp Code Editor:
Previous: Write a C# program to create a new string where the first 4 characters will be in lower case. If the string is less than 4 characters then make the whole string in upper case.
Next: Write a C# program to create a new string of every other character (odd position) from the first position of a given string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics