C#: Create a new string where the first 4 characters will be in lower case
First 4 Chars Lowercase, Rest Uppercase
Write a C# program to create a string where the first 4 characters are in lower case. If the string is less than 4 letters, make the whole string in upper case.
Pictorial Presentation:
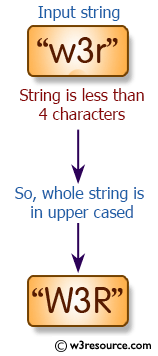
Sample Solution:
C# Sharp Code:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
public class Exercise42
{
public static void Main( )
{
// Prompt the user to input a string
Console.Write("Input a string: ");
// Read the input string and store it in the variable 'str'
string str = Console.ReadLine();
// Check if the length of the input string is less than 4 characters
if (str.Length < 4)
// If the length is less than 4, convert the whole string to uppercase and display it
Console.WriteLine(str.ToUpper());
else
// If the length is 4 or greater, convert the first 4 characters to lowercase
// and concatenate the rest of the string as is, then display the modified string
Console.WriteLine(str.Substring(0, 4).ToLower() + str.Substring(4, str.Length - 4));
}
}
Sample Output:
Input a string: w3r W3R
Flowchart:
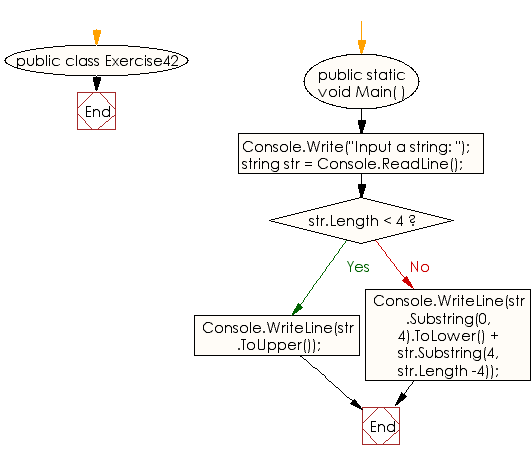
C# Sharp Code Editor:
Previous: Write a C# program to check if a given string contains 'w' character between 1 and 3 times.
Next: Write a C# program to check if a given string starts with "w" and immediately followed by two "ww".
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.