C#: Check if a given string contains 'w' character between 1 and 3 times
Check 'w' Appears 1-3 Times
Write a C# program to check if a given string contains the 'w' character between 1 and 3 times.
Pictorial Presentation:
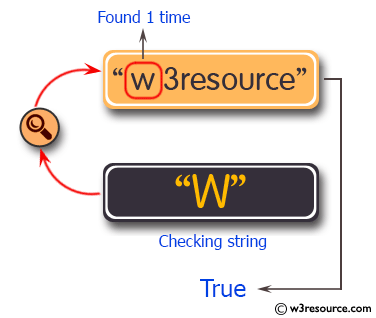
Sample Solution:
C# Sharp Code:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
public class Exercise41
{
public static void Main( )
{
// Prompt the user to input a string containing at least one 'w' character
Console.Write("Input a string (contains at least one 'w' char) : ");
// Read the input string and store it in the variable 'str'
string str = Console.ReadLine();
// Count the occurrences of the character 'w' in the input string and store the count in 'count'
var count = str.Count(s => s == 'w');
// Display a message indicating the test for the presence of 'w' character between 1 and 3 times
Console.WriteLine("Test the string contains 'w' character between 1 and 3 times: ");
// Check if the count of 'w' characters is between 1 and 3 (inclusive) and print the result
Console.WriteLine(count >= 1 && count <= 3);
}
}
Sample Output:
Input a string (contains at least one 'w' char) : w3resource Test the string contains 'w' character between 1 and 3 times: True
Flowchart:
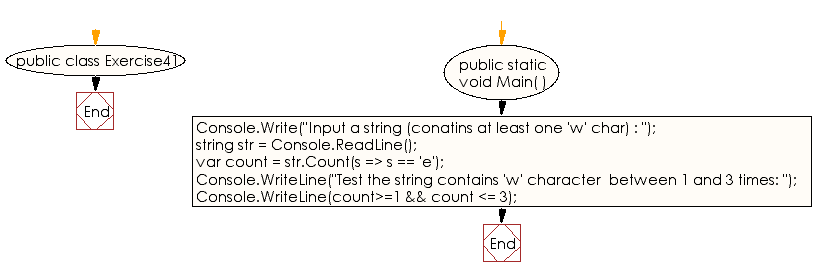
For more Practice: Solve these Related Problems:
- Write a C# program to count how many times 'w' appears in a string and return true if it's 1 to 3 times.
- Write a C# program to validate a string contains only one to three instances of any vowel.
- Write a C# program to return true if a string contains exactly 2 'w' characters.
- Write a C# program to find if a character appears between m and n times in a string.
Go to:
PREV : Nearest to 20 or Return 0.
NEXT : First 4 Chars Lowercase, Rest Uppercase.
C# Sharp Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.