C#: Check the nearest value of 20 of two given integers and return 0 if two numbers are same
Nearest to 20 or Return 0
Write a C# program that checks the nearest value of 20 of two given integers and return 0 if two numbers are same.
Pictorial Presentation:
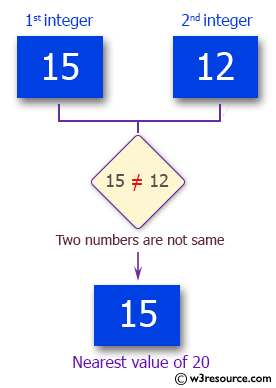
Sample Solution:
C# Sharp Code:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
public class Exercise40
{
public static void Main( )
{
// Prompt the user to input the first integer
Console.WriteLine("\nInput first integer:");
// Read the input and convert it to an integer 'x'
int x = Convert.ToInt32(Console.ReadLine());
// Prompt the user to input the second integer
Console.WriteLine("Input second integer:");
// Read the input and convert it to an integer 'y'
int y = Convert.ToInt32(Console.ReadLine());
// Declare and assign a constant integer 'n' with the value 20
int n = 20;
// Calculate the absolute difference between 'x' and 'n', and store it in 'val1'
var val1 = Math.Abs(x - n);
// Calculate the absolute difference between 'y' and 'n', and store it in 'val2'
var val2 = Math.Abs(y - n);
// Output 0 if 'val1' is equal to 'val2', otherwise output the number closer to 'n' among 'x' and 'y'
Console.WriteLine(val1 == val2 ? 0 : (val1 < val2 ? x : y));
}
}
Sample Output:
Input first integer: 15 Input second integer: 12 15
Flowchart:
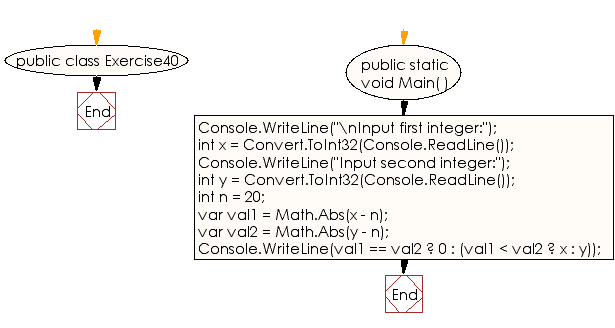
C# Sharp Code Editor:
Previous: Write a C# program to find the largest and lowest values from three integer values.
Next: Write a C# program to check if a given string contains 'w' character between 1 and 3 times.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.