C#: Find the largest and lowest values from three integer values
C# Sharp Basic: Exercise-39 with Solution
Write a C# program to find the largest and lowest values from three integer values.
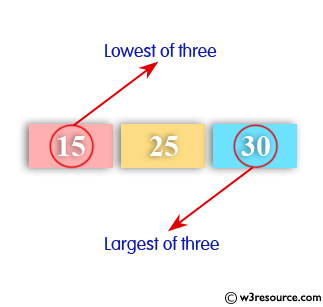
Sample Solution:
C# Sharp Code:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
public class Exercise39 {
static void Main(string[] args) {
// Prompt the user to input the first integer
Console.WriteLine("\nInput first integer:");
// Read the input and convert it to an integer 'x'
int x = Convert.ToInt32(Console.ReadLine());
// Prompt the user to input the second integer
Console.WriteLine("Input second integer:");
// Read the input and convert it to an integer 'y'
int y = Convert.ToInt32(Console.ReadLine());
// Prompt the user to input the third integer
Console.WriteLine("Input third integer:");
// Read the input and convert it to an integer 'z'
int z = Convert.ToInt32(Console.ReadLine());
// Find and display the largest of the three integers using Math.Max method
Console.WriteLine("Largest of three: " + Math.Max(x, Math.Max(y, z)));
// Find and display the smallest of the three integers using Math.Min method
Console.WriteLine("Lowest of three: " + Math.Min(x, Math.Min(y, z)));
}
}
Sample Output:
Input first integer: 15 Input second integer: 25 Input third integer: 30 Largest of three: 30 Lowest of three: 15
Flowchart:
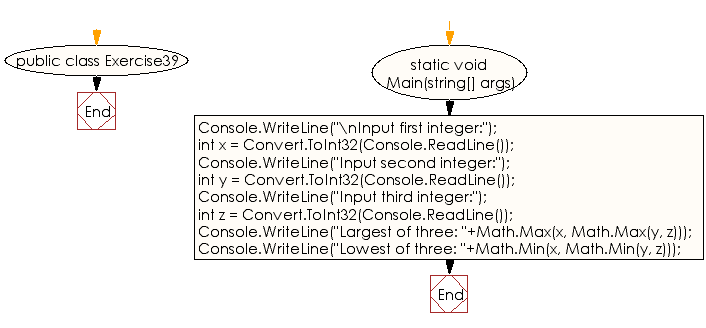
C# Sharp Code Editor:
Previous: Write a C# program to get a new string of two characters from a given string. The first and second character of the given string must be "P" and "H", so PHP will be "PH".
Next: Write a C# program to check the nearest value of 20 of two given integers and return 0 if two numbers are same.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/csharp-exercises/basic/csharp-basic-exercise-39.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics