C#: Get a new string of two characters from a given string
Extract 'PH' from String
Write a C# program to get a string of two characters from a given string. The first and second characters of the given string must be "P" and "H", so PHP will be "PH".
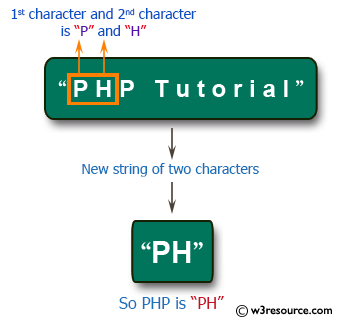
Sample Solution:-
C# Sharp Code:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
public class Exercise38 {
static void Main(string[] args) {
// Define a string variable 'str1' and assign it the value "PHP Tutorial"
string str1 = "PHP Tutorial";
// Initialize an empty string variable 'result' to store the concatenated characters
var result = "";
// Check if the length of 'str1' is greater than or equal to 1 and if the first character is 'P'
if (str1.Length >= 1 && str1[0] == 'P')
// If true, append the first character 'P' to the 'result' string
result += str1[0];
// Check if the length of 'str1' is greater than or equal to 2 and if the second character is 'H'
if (str1.Length >= 2 && str1[1] == 'H')
// If true, append the second character 'H' to the 'result' string
result += str1[1];
// Print the final concatenated string stored in the 'result' variable
Console.WriteLine(result);
}
}
Sample Output:
PH
Flowchart:
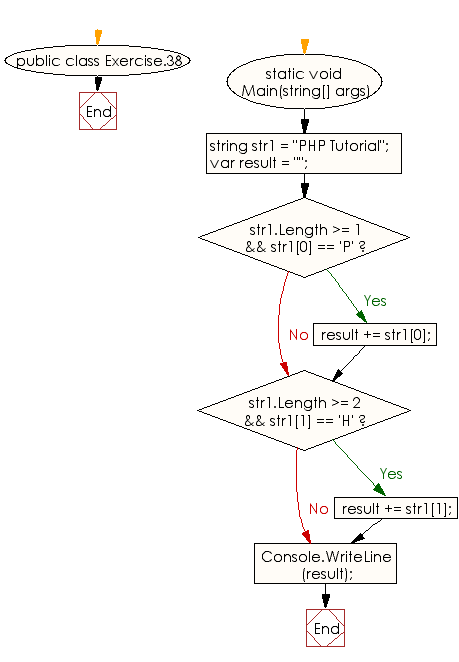
C# Sharp Code Editor:
Previous: Write a C# program to check if "HP" appears at second position in a string and returns the string without "HP".
Next: Write a C# program to find the largest and lowest values from three integer values.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics