C#: Check if an integer is in the range -10 to 10
Check Integer in Range -10 to 10
Write a C# program to check if an integer (from the two given integers) is in the range -10 to 10.
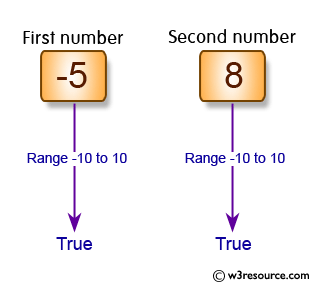
Sample Solution:-
C# Sharp Code:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
public class Exercise36 {
static void Main(string[] args) {
// Prompt the user to input the first number
Console.Write("Input a first number: ");
// Read user input and convert it to an integer 'm'
int m = Convert.ToInt32(Console.ReadLine());
// Prompt the user to input the second number
Console.Write("Input a second number: ");
// Read user input and convert it to an integer 'n'
int n = Convert.ToInt32(Console.ReadLine());
// Check if either the first number 'm' or the second number 'n' is within the range -10 to 10 (inclusive)
// Print the result of the logical OR operation between the conditions
Console.WriteLine(((m >= -10 && m <= 10)) || ((n >= -10 && n <= 10)));
}
}
Sample Output:
Input a first number: -5 Input a second number: 8 True
Flowchart:
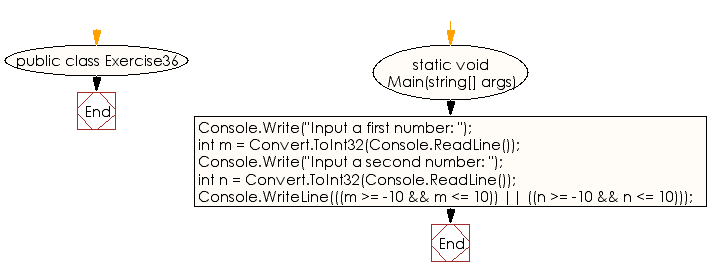
C# Sharp Code Editor:
Previous: Write a C# program to check two given numbers where one is less than 100 and other is greater than 200.
Next: Write a C# program to check if "HP" appears at second position in a string and returns the string without "HP".
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics