C#: Check if a given positive number is a multiple of 3 or a multiple of 7
Check Multiple of 3 or 7
Write a C# program to check if a given positive number is a multiple of 3 or 7.
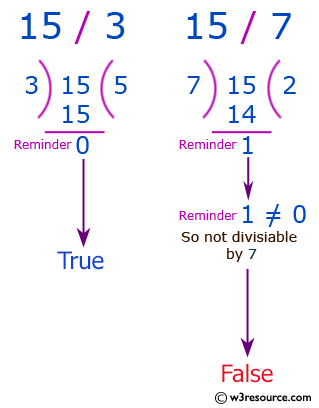
C# Sharp Code:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
public class Exercise33 {
static void Main(string[] args) {
// Display a message asking the user to input the first integer
Console.WriteLine("\nInput first integer:");
// Read the input from the user and convert it to an integer 'x'
int x = Convert.ToInt32(Console.ReadLine());
// Check if the entered number 'x' is greater than 0
if (x > 0) {
// If 'x' is positive, check if it is divisible by 3 or 7 using the modulo operator
// Print the result of the logical OR operation between the conditions (divisible by 3 or 7)
Console.WriteLine(x % 3 == 0 || x % 7 == 0);
}
}
}
Sample Output:
Input first integer: 15 True
Flowchart:
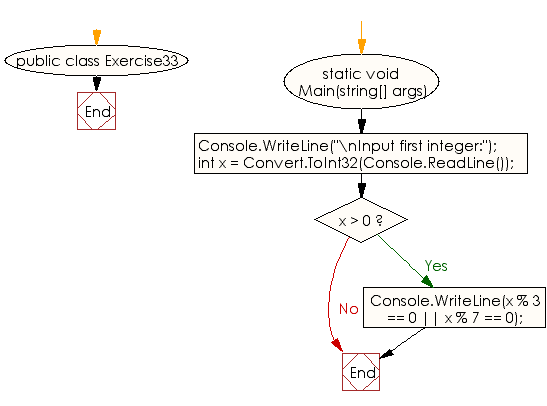
For more Practice: Solve these Related Problems:
- Write a C# program to find all numbers between 1 and 1000 which are divisible by both 3 and 7.
- Write a C# program to determine whether a given number is divisible by 3, 7, or both, and output a custom message.
- Write a C# program to check if a number is divisible by 3 or 7 but not both.
- Write a C# program to compute the sum of all numbers between 1 and n that are divisible by 3 or 7.
Go to:
PREV : Four Copies of Last Four Characters.
NEXT : Check String Starts with Word.
C# Sharp Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.