C#: Create a new string of four copies, taking last four characters from a given string
Four Copies of Last Four Characters
Write a C# program to create a string of four copies, taking the last four characters from a given string. If the given string is less than 4, return the original one.
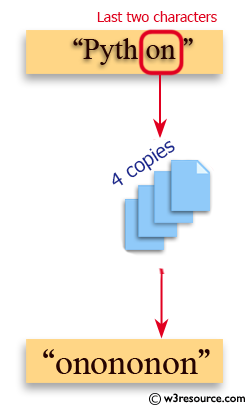
Sample Solution:-
C# Sharp Code:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
public class Exercise32 {
static void Main(string[] args) {
// Declare a string variable 'str' to store user input
string str;
// Declare an integer variable 'l' and initialize it to 0
int l = 0;
// Display a message asking the user to input a string
Console.Write("Input a string : ");
// Read user input and store it in the 'str' variable
str = Console.ReadLine();
// Check if the length of the input string is greater than 4
if (str.Length > 4) {
// If the length is less than 4, concatenate the input string thrice
// If the length is greater than or equal to 4, concatenate the last 4 characters of the input string four times
Console.WriteLine(str.Length < 4 ? str + str + str : str.Substring(str.Length - 4) + str.Substring(str.Length - 4) + str.Substring(str.Length - 4) + str.Substring(str.Length - 4));
}
}
}
Sample Output:
Input a string : The quick brown fox jumps over the lazy dog. dog.dog.dog.dog.
Flowchart:
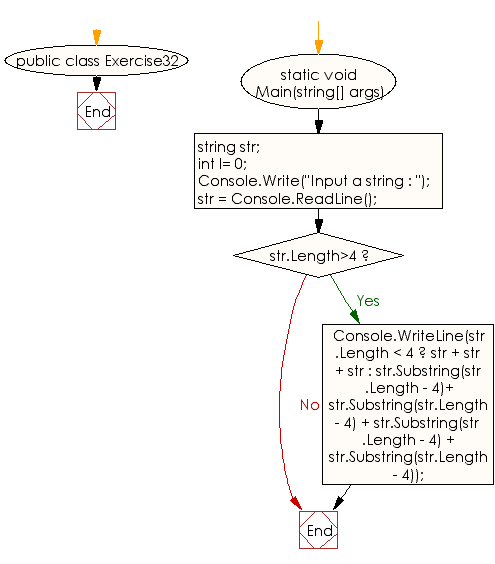
C# Sharp Code Editor:
Previous: Write a C# program to multiply corresponding elements of two arrays of integers.
Next: Write a C# program to check if a given positive number is a multiple of 3 or a multiple of 7.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.