C#: Multiply corresponding elements of two arrays of integers
Multiply Two Arrays
Write a C# program to multiply the corresponding elements of two integer arrays.
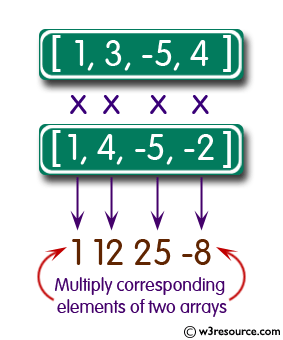
Sample Solution:-
C# Sharp Code:
using System;
using System.Collections.Generic;
public class Exercise31 {
public static void Main() {
// Declare and initialize the first array with integer values
int[] first_array = {1, 3, -5, 4};
// Declare and initialize the second array with integer values
int[] second_array = {1, 4, -5, -2};
// Display the elements of the first array
Console.WriteLine("\nArray1: [{0}]", string.Join(", ", first_array));
// Display the elements of the second array
Console.WriteLine("Array2: [{0}]", string.Join(", ", second_array));
// Display a message indicating multiplication of corresponding elements of both arrays
Console.WriteLine("\nMultiply corresponding elements of two arrays: ");
// Loop through the arrays to multiply corresponding elements and display the results
for (int i = 0; i < first_array.Length; i++) {
// Multiply the elements at index 'i' of both arrays and display the result
Console.Write(first_array[i] * second_array[i] + " ");
}
// Move to the next line for a clean output format
Console.WriteLine("\n");
}
}
Sample Output:
Array1: [1, 3, -5, 4] Array2: [1, 4, -5, -2] Multiply corresponding elements of two arrays: 1 12 25 -8
Flowchart:
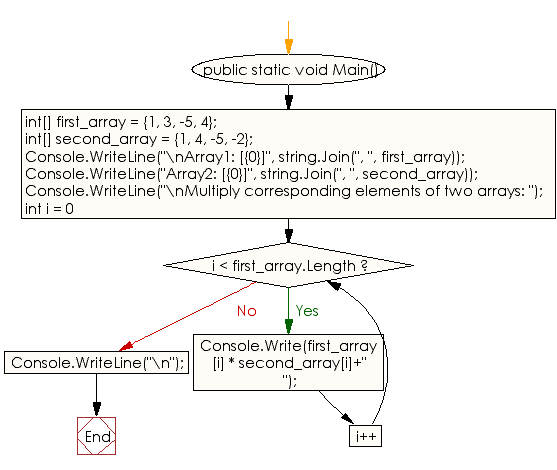
C# Sharp Code Editor:
Previous: Write a C# program to convert a hexadecimal number to decimal number.
Next: Write a C# program to create a new string of four copies, taking last four characters from a given string. If the length of the given string is less than 4 return the original one.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics