C#: Convert a hexadecimal number to decimal number
Hexadecimal to Decimal
Write a C# program to convert a hexadecimal number to a decimal number.
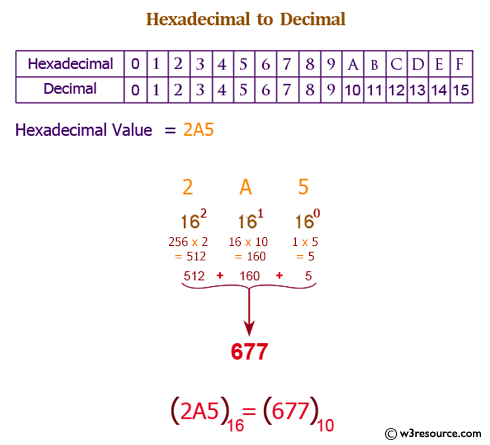
Sample Solution:-
C# Sharp Code:
using System;
using System.Collections.Generic;
public class Exercise30 {
public static void Main() {
// Declare a string variable 'hexval' and assign a hexadecimal value "4B0" to it
string hexval = "4B0";
// Display the original hexadecimal number
Console.WriteLine("Hexadecimal number: " + hexval);
// Convert the hexadecimal string 'hexval' to its decimal equivalent
int decValue = int.Parse(hexval, System.Globalization.NumberStyles.HexNumber);
// Display a message indicating conversion to different number systems
Console.WriteLine("Convert to-");
// Display the decimal value obtained from the hexadecimal conversion
Console.WriteLine("Decimal number: " + decValue);
}
}
Sample Output:
Hexadecimal number: 4B0 Convert to- Decimal number: 1200
Flowchart:
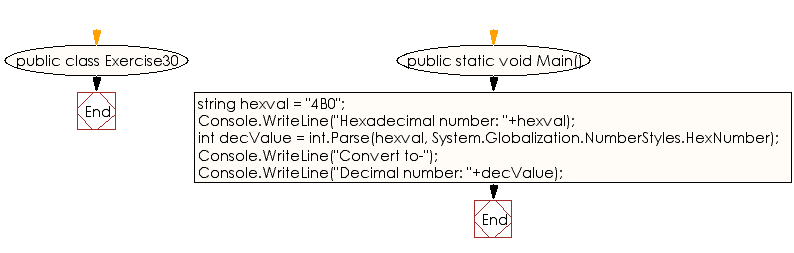
C# Sharp Code Editor:
Previous: Write a C# program to find the size of a specified file in bytes.
Next: Write a C# program to multiply corresponding elements of two arrays of integers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics