C#: Create a new string from a given string where the first and last characters will change their positions
Swap First and Last Characters
Write a C# program to create a new string from a given string where the first and last characters change their positions.
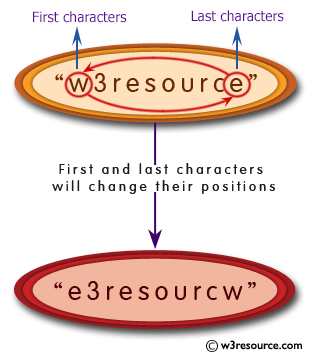
Sample Solution:
C# Sharp Code:
using System;
using System.Collections.Generic;
// This is the beginning of the Exercise16 class
public class Exercise16 {
// This is the main method where the program execution starts
static void Main(string[] args)
{
// Displaying the result of rearranging the first and last characters of a string
Console.WriteLine(first_last("w3resource")); // Rearranges the first and last characters of the string
Console.WriteLine(first_last("Python")); // Rearranges the first and last characters of the string
Console.WriteLine(first_last("x")); // Returns the same character for a single-character string
}
// Function to rearrange the first and last characters of a string
public static string first_last(string ustr)
{
// Using the ternary operator to rearrange characters based on the length of the string
return ustr.Length > 1
? ustr.Substring(ustr.Length - 1) + ustr.Substring(1, ustr.Length - 2) + ustr.Substring(0, 1)
: ustr; // Returns the same character for a single-character string
}
}
Test Data: w3resource
Sample Output:
e3resourcw nythoP x
Flowchart:
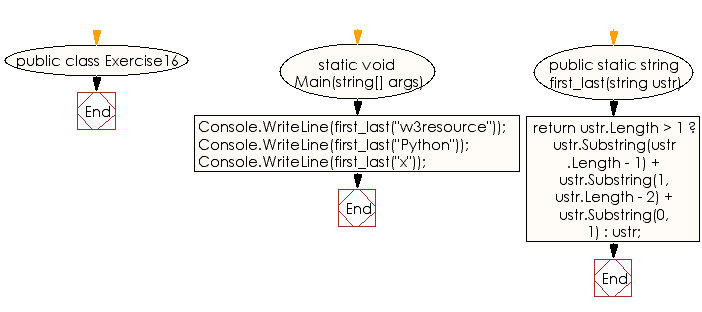
For more Practice: Solve these Related Problems:
- Write a C# program to swap the second and second-last characters of a string.
- Write a C# program to swap the first character with the middle character if the string length is odd.
- Write a C# program to perform a circular shift of characters: last becomes first, all others shift right.
- Write a C# program that swaps first and last characters only if the string length is greater than 3.
Go to:
PREV : Remove Character by Index.
NEXT : Add First Character to Front and Back.
C# Sharp Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.