C#: Remove specified a character from a non-empty string using index of a character
Remove Character by Index
Write a C# program that removes a specified character from a non-empty string using the index of a character.
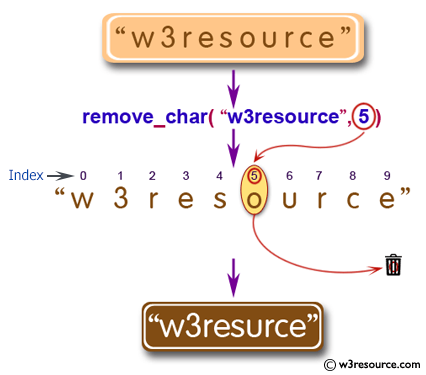
Sample Solution:
C# Sharp Code:
using System;
using System.Collections.Generic;
public class Exercise15 {
// This is the main method where the program execution starts
static void Main(string[] args)
{
// Displaying the result after removing a character at the specified index
Console.WriteLine(remove_char("w3resource", 1)); // Removes character at index 1
Console.WriteLine(remove_char("w3resource", 9)); // Removes character at index 9 (if exists)
Console.WriteLine(remove_char("w3resource", 0)); // Removes character at index 0
}
// Function to remove a character at the specified index
public static string remove_char(string str, int n)
{
return str.Remove(n, 1); // Using Remove method to eliminate the character at index n
}
}
Test Data: w3resource
Sample Output:
wresource w3resourc 3resource
Flowchart:
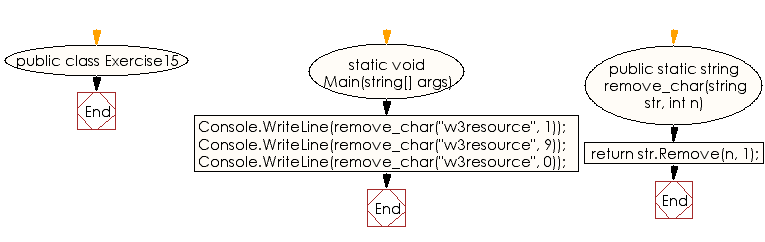
For more Practice: Solve these Related Problems:
- Write a C# program to remove the first and last characters of a string only if they are the same.
- Write a C# program that removes every third character from a given string using a loop.
- Write a C# program to remove characters from a string at all positions which are multiples of 3.
- Write a C# program that removes a character by index only if it is a vowel.
Go to:
PREV : Celsius to Kelvin and Fahrenheit.
NEXT : Swap First and Last Characters.
C# Sharp Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.