C#: Check whether a given array of integers of length 2, contains 15 or 20
Array of Length 2 Contains 15 or 20
Write a C# Sharp program to check if an array of integers length 2 contains 15 or 20.
Visual Presentation:
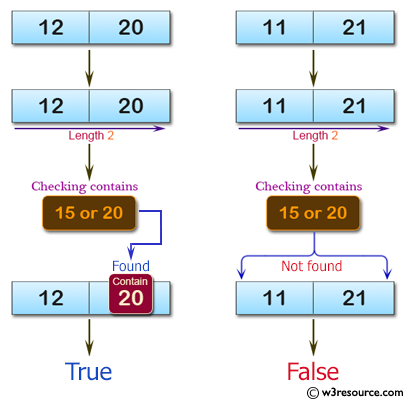
Sample Solution:-
C# Sharp Code:
using System; // Importing the System namespace
namespace exercises // Defining a namespace called 'exercises'
{
class Program // Defining a class named 'Program'
{
static void Main(string[] args) // The entry point of the program
{
// Output the result of the 'test' method with different integer arrays as arguments
Console.WriteLine(test(new[] { 12, 20 })); // Calling test method with array [12, 20] and displaying the result
Console.WriteLine(test(new[] { 14, 15 })); // Calling test method with array [14, 15] and displaying the result
Console.WriteLine(test(new[] { 11, 21 })); // Calling test method with array [11, 21] and displaying the result
}
// Method to check if any element in the input array is equal to 15 or 20
public static bool test(int[] nums)
{
// Returning true if any element in the array is equal to 15 or 20
return nums[0] == 15 || nums[0] == 20 || nums[1] == 15 || nums[1] == 20;
}
}
}
Sample Output:
True True False
Flowchart:
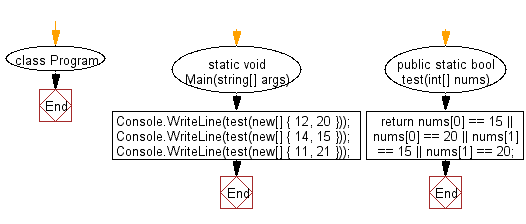
Go to:
PREV : First and Last Elements of Array.
NEXT : Array of Length 2 Does Not Contain 15 or 20.
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.